Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial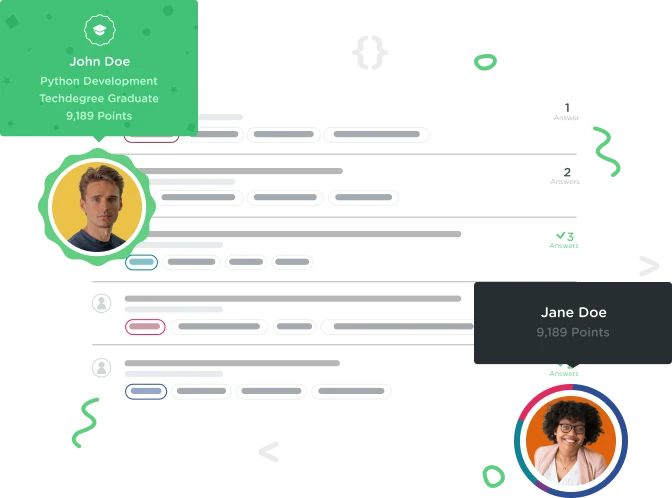

Tom Evertzen
9,750 PointsUsing a for in loop and not declaring the variable
I used a For in loop in my code, and everything is working like it should but i was wondering, 2 videos earlier there was specifically told in the teachers notes that we had to declare the variable in the for in loop. Like this for (var prop in students) but i noticed that when i do not declare that variable it is still working the same way, the chrome console and VSC aren't given any problems? Why is it still working and is it bad practice to leave it out?
var HTML = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
var students = [
{
name: 'Tom',
track: ['Javascript', 'JSON', 'CSS'],
achievements: ['HTML', 'Computeren'],
points: 1245
},
{
name: 'Eva',
track: ['Wordpress', 'Android', 'CSS'],
achievements: ['PHP', 'Computeren'],
points: 6543
},
{
name: 'Johnny',
track: ['Beginning PHP', 'Swift'],
achievements: ['HTML', 'Rails development'],
points: 23467
},
{
name: 'Kendrick',
track: ['Javascript', 'ASP.NET', 'Security'],
achievements: ['Design Primer', 'Java'],
points: 4312
},
{
name: 'Pietje',
track: ['C#', 'SQL', 'CSS'],
achievements: ['Exploring Flask', 'Beginning Python'],
points: 54346
}
];
var student;
for (prop in students) {
student = students[prop];
HTML += '</br>';
HTML += '<h2> Student: ' + student.name + '</h2>';
HTML += '<p>Track: ' + student.track.join(', ') + '.</p>';
HTML += '<p>Achievements: ' + student.achievements.join(', ') + '.</p>';
HTML += '<p>Points: ' + student.points + '.</p>';
}
print(HTML);
2 Answers
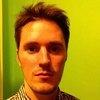
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThe difference is local vs global scope. If you happened to have another variable in the global scope called prop
, your loop would be modifying that variable every time. If you made your loop for (var prop in students)
or better yet for (let prop in students)
, then it would make a local variable that wouldn't pollute the global scope. So it's just good practice to keep the scope local just in case there was something floating around with the same name. Especially if you're collaborating with other devs, sometimes you don't actually know for sure what other variables might be out there in global scope.

Michel Ribbens
18,417 Pointsthis worked for me as well: for (prop in students)
am a bit confused with the ES6 way of writing variables in loops, arrays and objects. I want to make my code as modern as possible so instead of using var
I want to use const
or let
in the loop it seems to be an obvious choice since the variable is changing, so we should use let
but what about the other variables? so for example, here is my code for this challenge. please tell me when to use const
or let
instead of the old var
var text = '';
var student;
function print(message) {
var output = document.getElementById('output');
output.innerHTML = message;
}
for (prop in students) {
var student = students[prop];
text += `<h2><b>Student: ${student.name}</b></h2>`;
text += `<p>Track: ${student.track}</p>`;
text += `<p>Achievements: ${student.achievements}</p>`;
text += `<p>Points: ${student.points}</p>`;
text += `<br>`;
}
print(text);

Tom Evertzen
9,750 PointsThis should help Michel, at least for me it made it much clearer: https://teamtreehouse.com/library/welcome-to-getting-started-with-es2015
Tom Evertzen
9,750 PointsTom Evertzen
9,750 PointsThanks for the fast answer but i think i'm still not fully getting in haha. Is the for loop working the same as a function, and the parameters given in would automatically create a variable. No matter if it has let, const or var in front of it?
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsIt only creates a variable in local scope by default (like a function parameter) if you use the
let
keyword. If you don’t it looks for one in the global scope.Let me show you two examples. In both examples, I’m going to declare a global variable
number
. In the first example, I’m using thelet
keyword which means that in thefor in
loopnumber
will be a local variable that doesn’t affect the global scope. So you can see that I defined the number as “four” before the loop, and it’s still “four” after the loop.In another example, I don’t use the
let
keyword. So after the loop, if I print out the value ofnumber
, it’s “three”. So there has been a side effect of changing the global variable with that name as we went through the loop. Which could potentially cause bugs. So it’s better to just be in the habit of usinglet
.