Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial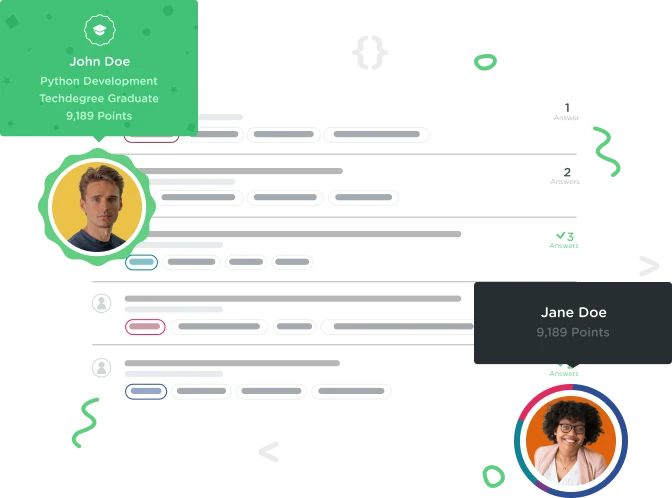

Rob Bartlett
6,741 PointsUsing a Function to Make This Cleaner
Ok, so I was able to pass this challenge by writing
var secret = prompt("What is the secret password?");
var entry = "sesame";
while (secret !== entry) {
secret = prompt("That is incorrect. What is the secret password?")
}
document.write("You know the secret password. Welcome.");
However, I had hoped to save myself the trouble of re-writing the variable and prompt by using a function (as well as exercise a more elegant solution). What I tried was:
function gatekeeper() {
var secret = prompt("What is the secret password?")};
var entry = "sesame";
gatekeeper ();
while (secret.toLowerCase !== entry) {
gatekeeper ();
}
document.write("You know the secret password. Welcome.");
I call gatekeeper before getting into the loop to establish var secret, but then once it gets to the while loop, I get the error that secret is undefined.
What gives?
4 Answers

Grove Software
1,508 PointsThe variable secret
is scoped to the gatekeeper
function, which means that anything outside the function can't see it. To be honest, even if you fixed that by declaring secret
outside of the gatekeeper
function, I'm not sure I'd call that more elegant solution.
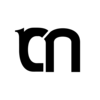
Colin Marshall
32,861 PointsThis is because of the variable scope of secret. You declared secret inside of the gatekeeper function. Since you did this, only the code inside of the function has access to that variable.

Rob Bartlett
6,741 PointsI figured that was the case. So, is there a way to make this work by just calling a function in the loop? Is the solution here simply to make the loop part of the function?

Jacob Mishkin
23,117 PointsThere are several things that this challenge is doing:
- understanding how the while loop works.
- understanding the conditional statement.
For number 1. from what you posted it seems like you understand this concept. While loop: While the condition is false do something.
For number 2. Let the conditional statement do the work for you. What I mean by that is, you don't need to set variables outside to set the condition to be true or false, that's the job of the statement itself. So in this case we are just checking if an input field equals a string, just add the string in the condition. No need for a new variable to be processed.
here is an example:
var secret = prompt("what is the secret password");
while (secret !== "sesame") {
secret = prompt("That is incorrect. What is the secret password?")
}
document.write("You know the secret password. Welcome.");
if you have any questions please ask.
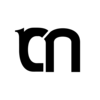
Colin Marshall
32,861 PointsOne issue I see with your example is that if you leave the secret variable empty when you declare it, you will be told you guessed incorrectly before you have had a chance to guess the password.

Jacob Mishkin
23,117 PointsColin, I see your point. I will make the changes. thanks.

Grove Software
1,508 PointsIf you did something like:
function gatekeeper() {
var secret = prompt("What is the secret password?");
var entry = "sesame";
return secret === entry;
}
while (! gatekeeper()) {
}
document.write("You know the secret password. Welcome.");
That might work. I'm not certain of how the while
loop would react to having a blocking operation in it like that.
Rob Bartlett
6,741 PointsRob Bartlett
6,741 PointsWell, more elegant in the sense that I'm avoiding repeating a line of code.
Grove Software
1,508 PointsGrove Software
1,508 PointsSometimes trying to avoid repeating yourself can be taken too far. You've ultimately repeated the variable in the form of calling
gateKeeper
multiple times.