Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial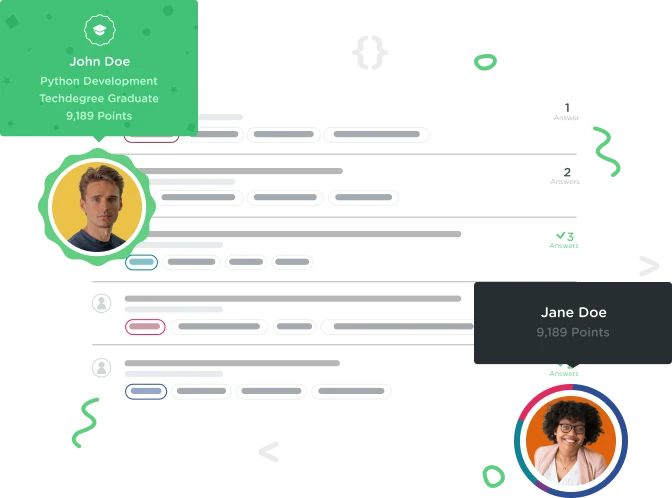

Erik Nuber
20,629 PointsUsing a hash symbol in the Balance Method
def addTransaction(description, amount)
@transactions.push(description: description, amount: amount)
end
def balance
balance = 0.0
@transactions.each do |transaction|
balance += transaction[:amount]
end
return balance
end
I am trying to figure out how transaction[:amount] accesses the amount in the array. I would have thought it would be transaction[amount]. I have been back thru the hash course and was unable to find anything other than a few seconds referring to :arg1 being a symbol and that there could be only one versus just arg1. But nothing else mentioned referencing hashes with the hash[:arg1] format.
below is the full code but, don't think it is necessary so I separated out the areas in question above.
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
addTransaction("Beginning Balance", 0)
end
def credit(description, amount)
addTransaction(description, amount)
end
def debit(description, amount)
addTransaction(description, -amount)
end
def addTransaction(description, amount)
@transactions.push(description: description, amount: amount)
end
def balance
balance = 0.0
@transactions.each do |transaction|
balance += transaction[:amount]
end
return balance
end
def to_s
"Name: #{name}, Balance: #{sprintf("%0.2f", balance)}"
end
def printRegister
puts "#{name}'s Bank Account"
puts "-" * 40
puts "Description".ljust(30) + "Amount".rjust(10)
@transactions.each do |trans|
puts trans[:description].ljust(30) + sprintf("%0.2f", trans[:amount]).rjust(10)
end
puts "-" * 40
puts "Balance:".ljust(30) + sprintf("%0.2f", balance).rjust(10)
puts "-" * 40
end
end
bank_account = BankAccount.new("Whomever")
bank_account.credit("Paycheck", 100)
bank_account.debit("Groceries", 40)
puts bank_account.printRegister
1 Answer

PoJung Chen
5,856 PointsThis is because we push hash key with symbol by
@transactions.push(description: description, amount: amount)
This is same as
@transactions.push({:description => description, :amount => amount})
Therefore, we can assess the value through @transaction[":description"]