Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial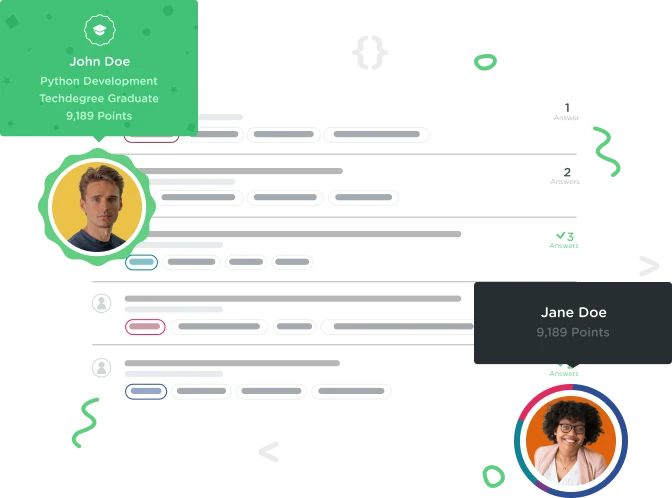

H Yang
2,066 PointsUsing a while/break loop
I've completed this challenge using the while, for, and do while loops. I'm trying to practice the break method for exiting the loop but I keep getting an error where the color is not concatenating and only prints out a single color. Can someone point me in the right direction?
var html = '';
var red;
var green;
var blue;
var rgbColor;
var counter = 0;
while (true) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
counter += 1;
if (counter = 10 ) {
break;
}
}
document.write(html);
Thank you.
2 Answers

Umesh Ravji
42,386 Pointsvar count = 3;
while (true) {
if (counter = 10) {
break;
}
}
console.log(counter); // 10
That is an assignment operation. The value of counter is being set to 10, and since that is truthy the if condition is true, and the break statement executes. So they loop only executes once, you get one item.
Compare that too:
var count = 3;
while (true) {
if (counter = 0) {
break;
}
}
If you set the counter to 0, the if condition becomes falsey and what you end up with is an infinite loop, so it's important to use comparison operators in this case.

H Yang
2,066 PointsArghh counter needs to be using a triple equals operator
var html = '';
var red;
var green;
var blue;
var rgbColor;
var counter = 0;
while (true) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
counter += 1;
if (counter === 10 ) {
break;
}
}
document.write(html);
Why is using the single one incorrect? Is using a single equals sign only for defining variables?

Umesh Ravji
42,386 PointsSingle equals is assignment operation, double equals for checking equality and triple is strict equality (or sometimes called identity, that looks at both value and type).
In this case the double equals should work too, but not the single.

David Bath
25,940 PointsYes, glad you figured it out on your own! A single "=" is called the assignment operator, which you use to assign a value to a variable. A double "==" and triple "===" are comparison operators.

H Yang
2,066 PointsThank you guys for the input. Having the proper terms helps a lot to keep track of the different operators.
So with that figured out, can either of you guys help me understand what's actually happening with my bugged code? What is actually happening in this
if (counter = 10 ) {
break;
}
that results in the html only getting one color value?

David Bath
25,940 PointsWhat's happening is that counter = 10
returns the value of counter, which is 10. That value is considered "truthy", and so the if
condition passes, and break
is called. So the html
variable only has the one color div at that point.

H Yang
2,066 PointsSo I wrote a true but irrelevant statement, the if condition was met, and the loop broke. Thanks for the insight David.
H Yang
2,066 PointsH Yang
2,066 PointsThank you. What you said about
counter = 10
being a true statement seems to make sense for me, but why is
counter = 0
a false statement?
David Bath
25,940 PointsDavid Bath
25,940 PointsHi Henry,
Do you know about "truthy" and "falsey" values in Javascript? I don't remember if it's covered in the JS courses on Treehouse, but you can probably find some information online. Here's the MDN documentation for false: https://developer.mozilla.org/en-US/docs/Glossary/Falsy
Basically, certain values can be interpreted as "false" values, and
0
is one of them!H Yang
2,066 PointsH Yang
2,066 PointsThanks a lot Umesh, David. I haven't yet covered truthy/falsey in this course yet but I'll do some reading here.