Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial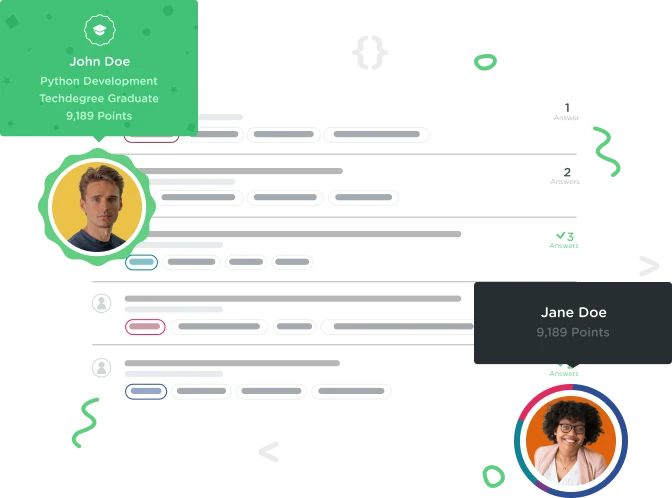
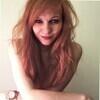
Silvia Ramos
Front End Web Development Techdegree Graduate 18,318 PointsUsing arrows
Hi there :)
Please, can someone check if this solution would be correct? I was using the arrows form before checking the teacher's solution, and looks like it works, but I am not sure if it would be a proper solution for this? Also, I am not sure either about using LET and CONST on this exercise.
Many thanks :))))
let getRandomNumber = (upper, lower) => {
const randomNumber = (Math.floor(Math.random() * (upper - lower + 1)) + lower)
return randomNumber
};
getRandomNumber(23, 8);
3 Answers

Guil Hernandez
Treehouse TeacherThat's absolutely correct. Nice work, @Silvia Ramos!
let
works in this case, and it's perfectly acceptable. Because you're assigning getRandomNumber
a function, it'd be safer to use const
to prevent the getRandomNumber
variable from being reassigned and losing the important functionality provided by getRandomNumber
:) For example,
let getRandomNumber = (upper, lower) => {
const randomNumber = (Math.floor(Math.random() * (upper - lower + 1)) + lower)
return randomNumber
};
getRandomNumber(23, 8); // 11
getRandomNumber = 5; // uh oh
getRandomNumber(100, 50); // Uncaught TypeError: getRandomNumber is not a function
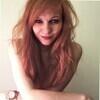
Silvia Ramos
Front End Web Development Techdegree Graduate 18,318 PointsHi Guil!
I see, thank you so much :)) makes sense to use CONST instead :) Thanks for your assistance :) As a note, you are being amazing throughout this course! (getting slow at understanding as my background on computers and programming was limited to switch on/off my laptop haha, but you make it 'easy'!) so glad I have joined TreeHouse!

Jason Cheung
3,227 Pointsjust wondering if I could simplify the code I have here.
function getRandomNumber(lower=1, upper=100) {
const randomNumber = Math.floor(Math.random() * (upper - lower + 1) ) + lower;
return randomNumber;
}
const lower = prompt("Enter the minimum number");
const upper = prompt("enter the maximum number");
if (Number.isInteger(+lower)===true){
if (Number.isInteger(+upper) === true){
alert(`${getRandomNumber(parseInt(lower), parseInt(upper))} is the random number between ${lower} and ${upper}`)
}else{
alert("Please enter numeric numbers")
}
}else{
alert("Please enter numeric numbers")
}
I have done much more than what it requested, but I think this is more interactive and can be an more user-friendly generator