Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial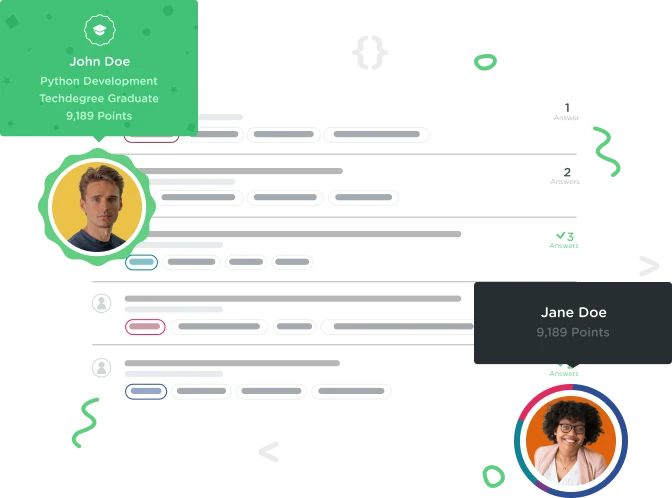
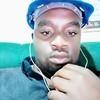
Dean Tinashe Katsaura
3,059 Pointsusing constructor to initialize field
Use constructor to initialize the TongueLength field to the value passed in
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tonguelength)
{
TongueLength = tonguelength;
TongueLength tonguelength = new TongueLength(tonguelength);
}
}
}
1 Answer
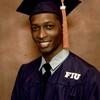
Dane Parchment
Treehouse Moderator 11,075 PointsThe problem here, is you are treating the value of the primitive data type (int) tongueLength as though it is a reference to a completely different class.
You have created primitive datatype variables before so how would you assign an integer value to a variable?
Like so correct?
int integerValue = 30;
So if you are told to assign the readonly integer variable TongueLength
an integer parameter you created in a constructor wouldn't you just assign TongueLength
to that parameter? You aren't referencing a new class so no need for the new className()
syntax. Also isn't it odd that there isn't a class called TongueLength
that was created for you to reference?
So to solve this problem just do the following:
class Frog
{
public readonly int TongueLength;
public Frog(int tonguelength)
{
this.TongueLength = tonguelength;
}
}
You were already so close! Keep at it, have fun programming and don't get discouraged, watch earlier c# videos if you aer having trouble remembering things like this.
Shlomi Bittan
6,718 PointsShlomi Bittan
6,718 PointsHi Dean, Do you have a class named 'TongueLength' that excepts int in its constructor? What is the error you get?