Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial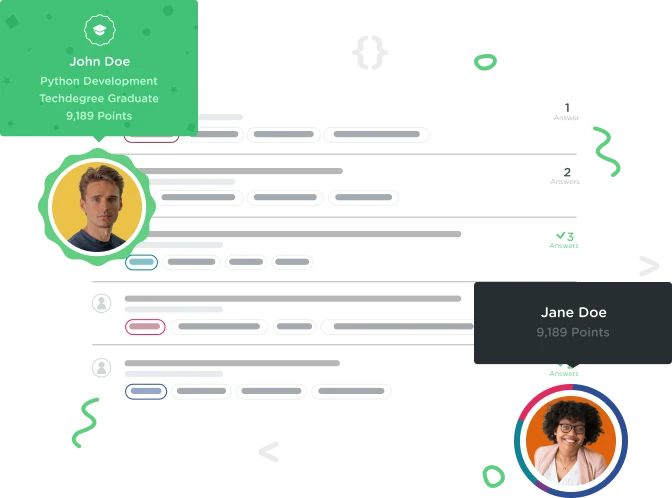

ishakahmed
11,359 PointsUsing current_user instead of g.user._get_current_object()
Would there be an error if I used current_user instead of g.user._get_current_object() here:
@app.route('/follow/<username>')
@login_required
def follow(username):
try:
to_user = models.User.get(models.User.username ** username)
except models.DoesNotExist:
pass
else:
try:
models.Relationship.create(
from_user=g.user._get_current_object(),
to_user=to_user
)
except models.IntegrityError:
pass
else:
flash("You're now following {}!".format(to_user.username), 'success')
return redirect(url_for('stream', username=to_user.username))
I am asking this because in the challenge following this video. I assigned current_user to from_user instead of g.user._get_current_object() and it wouldn't except my code. If there is a difference, what makes them different?
I wrote this:
@app.route('/follow/<int:user_id>')
@login_required
def follow(user_id):
models.Relationship.create(
from_user = current_user,
to_user = models.User.get(models.User.id == user_id)
)
return redirect(url_for('index'))
Instead of this (Right ANSWER):
@app.route('/follow/<int:user_id>')
@login_required
def follow(user_id):
models.Relationship.create(
from_user = g.user._get_current_object(),
to_user = models.User.get(models.User.id == user_id)
)
return redirect(url_for('index'))
1 Answer

Josh Keenan
20,315 PointsThey are different, g.user
is something that is available for the lifetime of the current request, and at the end of it, terminates (after creating the relationship).
current_user
on the other hand, is available for as long as a session is maintained (as long as the user is logged in). The two may look the same but the mechanisms running behind them are not, hope this helps and feel free to ask any questions.
ishakahmed
11,359 Pointsishakahmed
11,359 PointsThanks for the reply! I have a follow up question. If the difference between the two is length it's available, why does one cause an error (current_user) and not the other? This is even more confusing because g.user._get_current_object is a reference to current_user for its lifetime.
Josh Keenan
20,315 PointsJosh Keenan
20,315 PointsIt's attached to the request, it isn't that they survive longer but one is accessed from the request, when that ceases it is gone, so once a request is made that flies in and out straight away. But you put it well, it is a reference to current_user for its lifetime BUT it is attached to a request, it isn't available outside of that, it isn't a factor of 'length' of life, but the source of it. One is essentially a huge flask global, the other is a huge flask global only available on a request.