Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial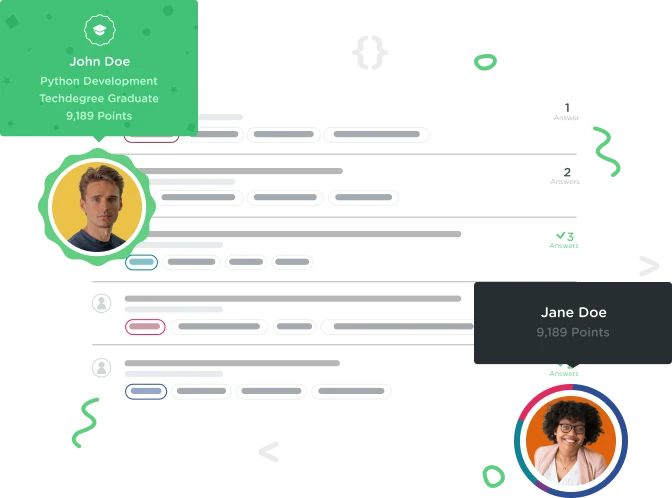

Ruben Corvalan
782 PointsUsing dictionary with an array.
I would like to access the values of an array inside a Dictionary using a tuple. In this particular case I would like to access the value of the array for the key "Ruben" by assigning the array value of "Ruben" to the tuple and then prince the array values.
Here it is.
var dictionary: [String:Double]
dictionary = ["Ruben": [1952.45, 56.87], "Peter": [456.34, 98.98]]
let tuple: (Double, Double)
tuple = dictionary["Ruben"]
The error at the last line is "Cannot assign value of type '[Double]?' to type '(Double, Double)'"
I think I am misunderstanding how can I assign a dictionary array to a tuple.
Please help.
Thanks.
2 Answers

Dhanish Gajjar
20,185 PointsThe way that the dictionary is defined is incorrect. Since the value in your <key, Value> Pair of dictionary is an Array of Doubles, the correct definition would be
var dictionary: [String: [Double]]
dictionary = ["Ruben": [1952.45, 56.87], "Peter": [456.34, 98.98]]
To access the value of any given key, the implementation is simple
let value = dictionary["Ruben"]
Hope it helps.

Dhanish Gajjar
20,185 PointsRuben Corvalan If you haven't finished Enumerations and Optionals course, then it will be confusing, I suggest you go through videos in the courses, wait till you get to that point.
There is a concept of optional binding that you will learn in Enumerations and Optionals course.
let dictionary = ["Ruben": [1952.45, 56.87], "Peter": [456.34, 98.98]]
if let value = dictionary["Ruben"] {
let singleValue = value[0]
}
All it says is we are assigning the dictionary value from <key, value> pair of the dictionary to a constant named value. So now the Array of Doubles is assigned to that constant. We can now used value[0] to retrieve the first value in the array and value[1] to retrieve the second value.
Ruben Corvalan
782 PointsRuben Corvalan
782 PointsGreat, thanks. And how can I access a single value of the available values inside the array? Examples, how can I access the double 56.87 in the key "Ruben"? Thanks.