Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial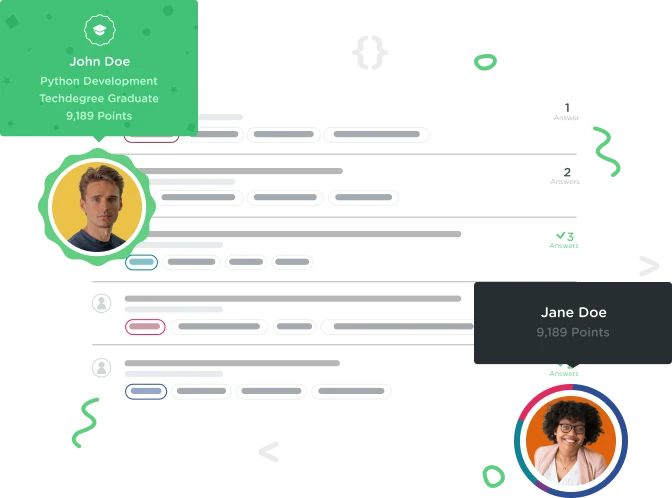

McKenzie Klasing
1,720 PointsUsing Dot Notation to access an attribute in class Panda - receiving: NameError: name 'Panda' is not defined
This is my first time asking a question so I apologize if it isn't formatted correctly.
This Challenge task reads as follows:
Create a method called eat
. It should only take self
as an argument. Inside of the method, set the is_hungry
attribute to False
, since the Panda will no longer be hungry when it eats. Also, return a string that says ''Bao Bao eats bamboo.'' where ''Bao Bao'' is the name attribute and ''bamboo'' is the food attribute.
I receive this error: NameError: name 'Panda' is not defined
regarding line 12, return str(f'{self.name} eats {Panda.food}')
I am able to get this code to run in my own IDE (mu) and it prints "Bao Bao eats bamboo" which tells me Panda.food
is working properly. I've tried numerous things to fix this like using self.food
but of course that doesn't work because this is a class attribute, not an instance attribute... Am I getting this wrong or is the task grader/checker looking for it to be written a different way?
class Panda():
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return str(f'{self.name} eats {Panda.food}')
panda_one = Panda('Bao Bao', 4)
print(panda_one.eat())
3 Answers
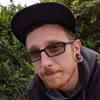
Travis Alstrand
Data Analysis Techdegree Graduate 45,998 PointsHey there McKenzie Klasing !
So one very sneaky thing I just noticed was at one point I had to look in the Test Results at the error and it mentions to "make sure there is a period".
We also don't need to use the str()
method, we can just straight up return the f-string and use self.food
return f'{self.name} eats {self.food}.'

Chuwen Tan
5,786 PointsI would like to know for this question, since food is a class attribute, not an instance attribute. Can I write
return f'{self.name} eats {Panda.food}.'
? Thank you!
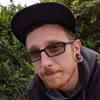
Travis Alstrand
Data Analysis Techdegree Graduate 45,998 PointsOut in the real world, yes you can. I just copied the code over to a new Python file in my local text editor and it printed the statement just fine in the console.
This challenge is just looking for self.food
specifically and will throw a regex related Assertion Error unfortunately if we try Panda.food
.
Here's what I ran locally though if you wanted to see for yourself
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return f"{self.name} eats {Panda.food}."
new_panda = Panda("Taco", 75)
print(new_panda.eat())

Chuwen Tan
5,786 PointsThank you for the elaboration Travis Alstrand!
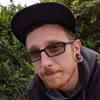
Travis Alstrand
Data Analysis Techdegree Graduate 45,998 PointsYou're very welcome!
McKenzie Klasing
1,720 PointsMcKenzie Klasing
1,720 PointsAwesome thanks Travis!
That did work! I also had to remove the print statement at the end. I appreciate you taking the time to assist me with this-
Travis Alstrand
Data Analysis Techdegree Graduate 45,998 PointsTravis Alstrand
Data Analysis Techdegree Graduate 45,998 PointsYaay! I'm glad it worked out and you got through it!