Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial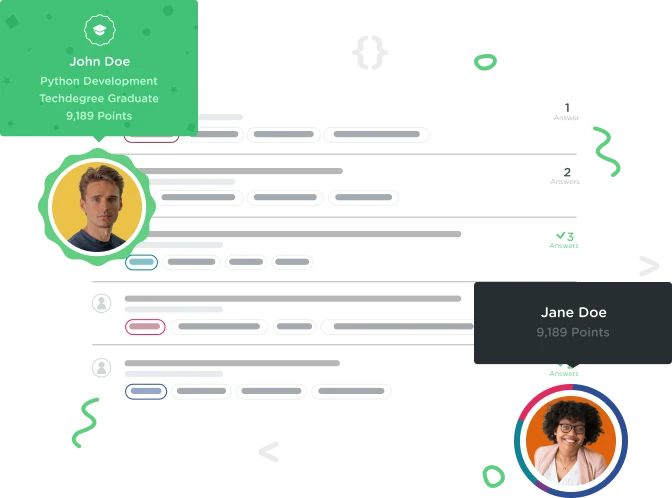
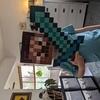
Gabbie Metheny
33,778 PointsUsing Existing Files from React Components Course
In case anyone wants to use their existing files from the React Components course, they should be virtually the same as the project files for React Context API. It looks like Guil has removed the high score functionality we built in the last couple videos, so you might have to work around that when managing state, and he also componentized PlayerList
instead of mapping through the players
array directly in App's render
method.
My final code for React Components is here, and my Context API "starter files" (that are functionally similar to Guil's) are here, in case that helps anyone else modify their existing project!
App.js
// import PlayerList instead of Player
import PlayerList from './PlayerList';
class App extends Component {
...
render() {
return (
<div className="scoreboard">
<Header players={this.state.players} />
<PlayerList
players={this.state.players}
changeScore={this.handleScoreChange}
removePlayer={this.handleRemovePlayer}
{/* Guil doesn't have this line, but I left it in the from React Components course */}
getHighScore={this.getHighScore}
/>
<AddPlayerForm addPlayer={this.handleAddPlayer} />
</div>
);
}
}
PlayerList.js
import React from 'react';
import Player from './Player';
const PlayerList = ({players, getHighScore, changeScore, removePlayer}) => {
return (
<div>
{players.map((player, index) =>
<Player
index={index}
key={player.id.toString()}
id={player.id}
name={player.name}
score={player.score}
changeScore={changeScore}
removePlayer={removePlayer}
{/* like in App.js, Guil does not have the high score functionality built in the previous course */}
isHighScore={getHighScore() === player.score}
/>
)}
</div>
);
};
export default PlayerList;
I'll update here if I run into any big issues reusing the old code, and I'll continue updating with new branches on Github for each video. Hope it helps someone!
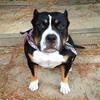
Mark Warren
19,252 PointsI agree. Thanks for this.
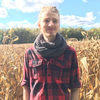
Andrew Bruner
Full Stack JavaScript Techdegree Graduate 27,932 PointsWow! Thanks again!
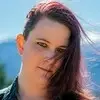
Jessie Starborne
6,875 PointsThank you from 2021!
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsGreat post Gabbie, thanks for providing it! :)