Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial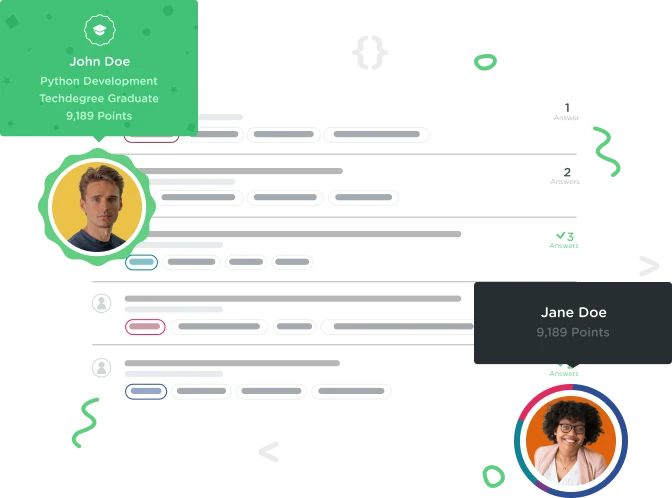

Arius Eich
2,769 PointsUsing extensions
I do not understand how to execute the question as well as how to execute it. Are they asking to add an integer to a string as in just place it in there or do they want the number added to the number already in the string? This is the objective: Your task is to extend the String type and add a method that lets you add an integer value to the string. For example: "1".add(value: 2) If the operation is possible, return an integer value. If the string does not contain an integer and you cannot add to it then return nil. Thank you for any help!
// Enter your code below
extension String {
var add: var(value: string) {
if value == 0 {
return nil
if else {
return
}
}
}
}
1 Answer

Magnus Hållberg
17,232 PointsThey are asking you to extend the String type with a method that checks if the string is a number (specifically an Int), if it is you are supposed to add that to the input from the method. Hope that makes sense. Since the method should return nil if the string is not an Int the return must be optional. This is one way of solving it.
extension String {
func add(value: Int) -> Int? {
// check if the string is an Int
if let stringInt = Int(self) {
// if the string is an Int, add the value from the method input and return it
return stringInt + value
} else {
// if its not an Int, return nil
return nil
}
}
}
Using the extension could look something like this.
let myString = "2"
myString.add(value: 2)
// myString is now of the type optional Int with the value 4
let myString2 = "Hi"
mystring2.add(value: 2)
// myString2 is now of the type optional Int with the value nil
Hope this helps