Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial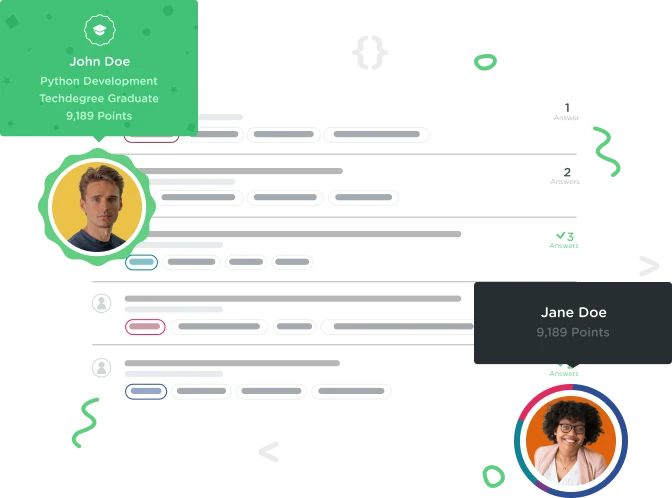

Daniel Spearman
6,640 PointsUsing "for in" loop in objects. Using "var" for the variable.
Sorry if this is newbie kind of question.
I noticed in the video that "var" was never placed with the variable in the following code:
var person = { name : 'Sarah', country : 'US', age : 35, treehouseStudent : true, skills : ['JavaScript', 'HTML', 'CSS'] };
for (prop in person){
console.log(prop, ': ', person[prop])
}
However, when taking the objective quiz, it specifically asked for me to specify that it was a variable, like so:
var shanghai = { population: 14.35e6, longitude: '31.2000 N', latitude: '121.5000 E', country: 'CHN' };
for (var prop in shanghai){ console.log(prop); }
I am still very new to this and want to make sure I dont add to my confusion down the road.
1 Answer

Wiktor Bednarz
18,647 PointsHey Daniel,
whenever you create a new named variable, will it be served globally or in some scope, like function, then it should always be declared with var (but prefferably with const or let - but I guess you didn't learn about ES6 yet). However it doesn't mean that this line of code will not work:
for (prop in person) {
// do something
}
In this case you create a variable, and since it's not declared the JavaScript interpreter just hoists prop into the global scope. So in short it assigns it as a variable of global scope for you. This is however a bad practice and will throw you a syntax error if you run this same line like this:
'use strict';
for (prop in person) {
// do something
}
This is because using this strict mode doesn't allow you to introduce those minor and most importantly silent errors into your application. They are silent because they won't be noticed on their own when first encountered in the application. The problem would be noticed only if you would use the same variable again (prop) in your application and it was also assigned to a global scope e.g:
const someFunction = person => {
for (prop in person) {
// do something
}
// return something
}
const otherFuncton = animal => {
for (prop in animal) {
// do something different
}
// return something different
}
The above code would treat the prop variable for two functions as the same one.
So in the end make sure to always declare your variables with var, let or const. I'll once again mention that var declaration has become pretty much obsolete with ES6, and using it is considered more or less as a bad practice even though it gets the job done.
I hope this sheds some light on your question,
Wiktor Bednarz
Daniel Spearman
6,640 PointsDaniel Spearman
6,640 PointsThank you very much for clarifying!