Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial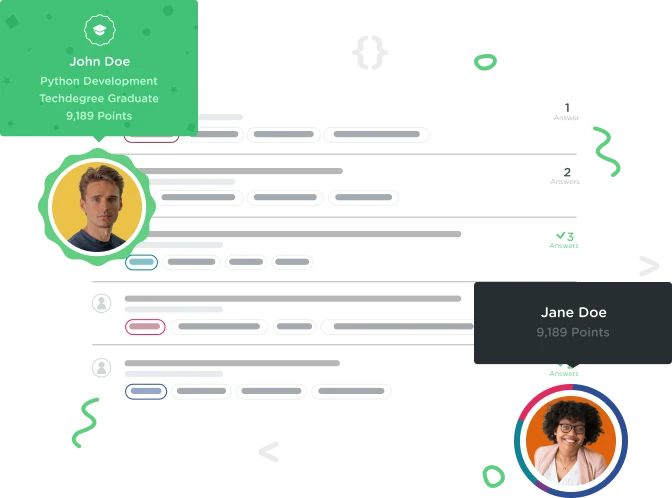

Greg Schudel
4,090 PointsUsing For Loops to Access an array
Got some questions about how a function interacts with an array. My questions are within the code...
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
function print(message) {
document.write(message);
/*the argument name must corrolate with the method that is used in the function*/
}
function buildList(list){ /*the argument list is passed into the function, what in the the function tells it we are minipulating the playlist?
Shouldn't there be a word in there that matches playList?*/
listHTML = '<h2>'; /*Why is it okay to use a '+=' operator here but not on the same variable with the closing tag below?*/
for (var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
/*The [i] runs through the entire array list*/
}
listHTML += '</h2>';
print(listHTML); /*why would you print it inside the function? Won't that make it local instead of global?
Don't you want it global so you can call it?*/
}
buildList(playList);
4 Answers
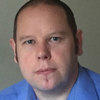
Alan McClenaghan
Full Stack JavaScript Techdegree Graduate 56,500 PointsHi Greg,
I'm trying to get to grips with this myself and, like you I think its finally beginning to sink in.
Rather than telling you how it is from the POV of an expert, here's how I am interpreting it as a learner:
The key part in this seems to be when the function is called: printList(playList);
. The array is passed into the function via the argument playList
which takes the place of the list
parameter in function printList( list )
. list.length
effectively becomes playList.length
and list[i]
becomes playList[i].
Similarly print(listHTML);
calls the print(message)
function and replaces the message
parameter with the argument listHTML
, which was the variable created inside the printList( )
function.
It might have been easier to understand if the message
and list
parameters inside the function parentheses had said listHTML
and playList
instead, but it really doesn't matter as they are basically only placeholders for the arguments that are passed into the functions when they are called.
Here's how it could be rewritten, with comments added about what I believe is happening:
//Loops through an array called playList and prints each item to the page.
//Array called playlist containing a list of songs
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
//Function called print with a parameter of listHTML (but this can be anything: an argument
will be added when the function is called)
function print(listHTML) {
document.write(listHTML);//Prints listHTML to the page when the functions is called.
}
//Function called printList with a parameter of playList (but this can be anything: an argument
will be added when the function is called)
function printList(playList) {
var listHTML = '<ol>';//Create a variable called listHTML with the value of an opening ol tag
for ( var i = 0; i < playList.length; i += 1) {//Loops through the playList parameter set above
listHTML += '<li>' + playList[i] + '</li>';//Concatenates a list using the items in parameter
set above.
}
listHTML += '</ol>';//Adds an ending ol tag to the listHTML variable
print(listHTML);//Calls the print() function declared above and passes listHTML variable
as an argument
}
printList(playList);//Calls the printList() function and passes it the playList array as an argument.
This creates the list and prints it to the page.
Expert rebuttals/corrections welcome.

Greg Schudel
4,090 PointsGreat! Much more clearer.
what is the purpose of the 'list[i]' within the function? I know it runs through the array, but why can't I use that to run through the entire array and print it out outside the function?
I think I will review the functions and conditionals to ensure I understand them as clearly as I should.

Greg Schudel
4,090 Pointsokay, I reviewed the videos, I understand why 'list[i]' is used in for loops for run through the array. I guess I didn't have the light go off as to what it was for until I reviewed for it.
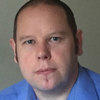
Alan McClenaghan
Full Stack JavaScript Techdegree Graduate 56,500 PointsHi Greg,
Looks like you got it already, but if only to help deepen my own understanding here is another go at explaining list[i]:
list
refers to the parameter set in the function declaration function buildList(list)
.
list
has no meaning in itself but is a placeholder for the argument to will be passed to it when the function is called later using buildList(playList)
.
list
now holds the playList array.
In the for loop, list.length now refers to the number of songs in the playList array, which is 6.
It loops through the array adding <li>
tags to create a formatted HTML list of 6 songs for when the list is printed to the page by the print(listHTML)
function.
The i
in the loop is also a placeholder that starts off at position 0 ('I Did It My Way') and then incrementally loops through the array to position 5 ('Maybelline').
So each time through the loop, the function looks at the playList and references the song at the appropriate position.
I hope that helps. It certainly helps me to explain it to myself.
As part of my own study, I've started keeping .js files containing notes explaining the theory and examples of code that I've gone through and commented in detail explaining to myself what each line is doing and how it relates to the whole.
This is very enlightening and its helping me to better understand what I'm doing.
Mark Miller
45,831 PointsMark Miller
45,831 PointsThe word "list" is just a prototype, so the actual thing passed in is playList in this example, as it says at the bottom of the file, after this function's prototype is finished being written. On the last line in file - buildList(playList) is the use of the function, so the declaration is using (list) but it could say anything there for declarative purposes only. So, playList is represented by the word "list" in the declaration. It's called a prototype or a declaration, the actual use of the function is called for at the end of the file, and playList is passed in. You can name yours "anyListPassedIn" to make it more clear.
The += is a way of building the string's value, concatenating the new assignment to the already existing string.
The += is also used in the for() condition to increment the variable i by one. It's a popular shorthand in most languages, meaning i = i + 1.
The print line will produce a return value that is output to anywhere of your choice when the function is called. Well it will return the list in the location that the function is used in any program. It's used at the end of this file, so it's outside of the function there.