Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial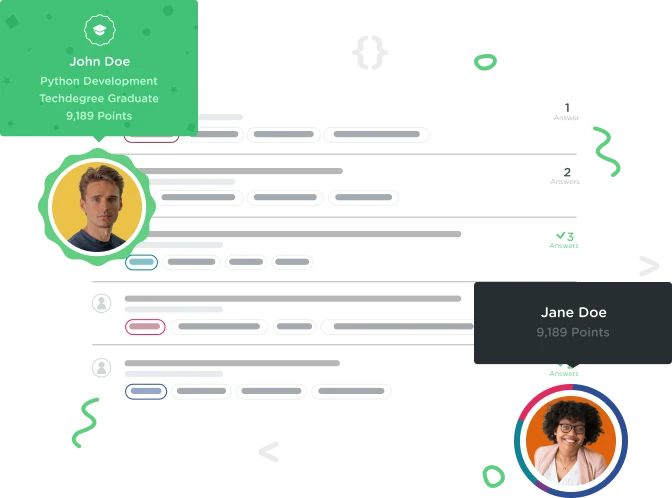
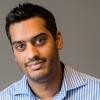
Jiten Mehta
Front End Web Development Techdegree Graduate 21,209 Pointsusing forEach instead of Reduce
Hey everyone, quick question.
Before Guil used the Reduce method to get a total player score, I paused the video and tried to do it myself. The solution I came up with was to use a forEach method.
It seems to work, but just wanted to ask if this method is still a good way of getting a totalScore or would it be more beneficial to use the Reduce method as Guil did?
Code below. Thanks!
const Stats = (props) =>{
const totalPlayers = props.players.length;
let totalScore = 0;
props.players.forEach(player => {
totalScore = totalScore += player.score;
});
return(
<table className="stats">
<tbody>
<tr>
<td>Players:</td>
<td>{totalPlayers}</td>
</tr>
<tr>
<td>Total Points:</td>
<td>{totalScore}</td>
</tr>
</tbody>
</table>
1 Answer
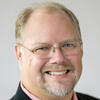
Jason Larson
8,353 PointsforEach() works for this, but most devs will tend toward another method for readability because you can define the expression outside of the method (as in let totalScore = ...
), whereas you can't do that with forEach() because forEach always returns undefined. It would also depend on whether or not you were doing anything else with the data, as you cannot chain to forEach(), but you can chain with other methods (like map()).
This isn't directly related to your question, but I thought I would mention that your expression for calculating the totalScore, while it does work as it is written, is very bad form. It should be either totalScore += player.score
or totalScore = totalScore + player.score