Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial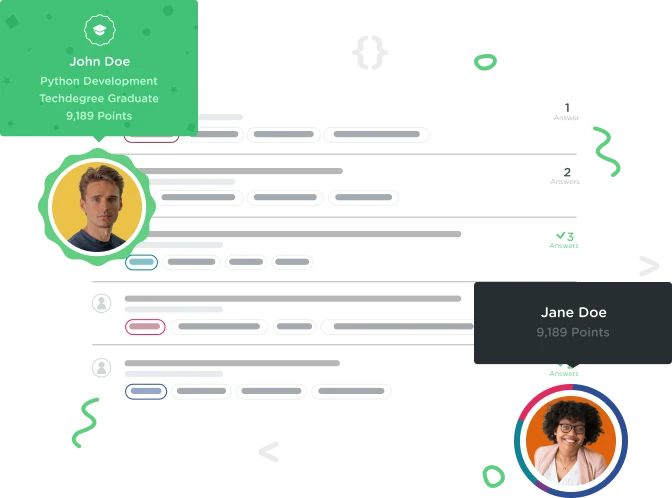

Homa Shayan
2,038 Pointsusing groupdict() method to pass re.search patterns into a class
Wow! OK, now, create a class named Player that has those same three attributes, last_name, first_name, and score. I should be able to set them through init.
So I'm using the groupdict() on my players (list? tuple? I'm actually not sure what re.search gives us) . Not sure what is exactly wrong.
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r'''
(?P<last_name>[\w\s]+),\s
(?P<first_name>[\w\s]+):\s
(?P<score>[\d]+)
''', string, re.X| re.M)
class Player:
def __int__(self, **players.groupdict()):
self.last_name = players.groupdict('last_name')
self.first_name = players.groupdict('first_name')
self.score = players.groupdict('score')
1 Answer

Dan Johnson
40,533 Pointssearch returns a match object, which is why it has the groupdict method.
For your class definition there's a few things. For the magic method:
def __int__(self, **players.groupdict()):
You were probably looking for __init__
instead of __int__
. __int__
also happens to be a magic method, but it's for how you deal with conversions to an int.
Another thing is that since you're defining the function you can't put **players.groupdict()
in the signature, you just want to define a name for that argument. You will, however, want to define the __init__
method in such a way that you could unpack the result of groupdict in order to construct a Player
, like this:
# ...
class Player(object):
def __init__(self, last_name, first_name, score):
# Do all the assignment here. No call to groupdict
# is required.
# You don't need this line for the challenge.
# This is just to show how you could create
# a new player with groupdict.
example = Player(**players.groupdict())