Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial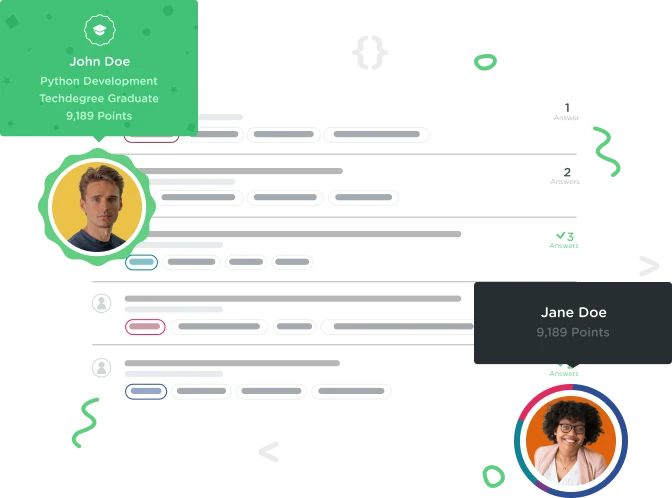
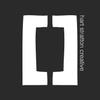
Angelica Hart Lindh
19,465 PointsUsing Gulp.js instead of webpack to split vendor and app scripts
Wanted to have a go at splitting the vendor and app scripts using Gulp.js instead of Webpack. For those of you who would prefer using Gulp, this is my solution:
'use strict';
var gulp = require('gulp');
var browserify = require('browserify');
var source = require('vinyl-source-stream');
var map = require('gulp-sourcemaps');
var packageJSON = require('./package.json');
var dependencies = Object.keys(packageJSON && packageJSON.dependencies || {});
gulp.task('vendor', function() {
return browserify()
.require(dependencies)
.bundle()
.pipe(source('vendor.bundle.js'))
.pipe(gulp.dest(__dirname + '/public/scripts'));
});
gulp.task('todo', function() {
return browserify('app/scripts/app.js')
.external(dependencies)
.bundle()
.pipe(source('todo.bundle.js'))
.pipe(map.write('./'))
.pipe(gulp.dest(__dirname + '/public/scripts'));
});
gulp.task('watch', function() {
gulp.watch('package.json', ['vendor']);
gulp.watch('app/scripts/**', ['todo']);
});
gulp.task('default', ['vendor', 'todo', 'watch']);
3 Answers

Steven Stanton
59,998 PointsCool, thanks :)
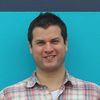
Sergio Alen
26,726 PointsThis is excellent, thanks! First thing that cross my mind was why use webpack when we can use Gulp.js or Grunt.js which are almost a must use already when developing this kind of applications.
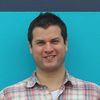
Sergio Alen
26,726 PointsAlthough I appreciate the teacher showing us another method.
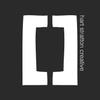
Angelica Hart Lindh
19,465 PointsHi Sergio,
Since posting this example where I used Gulp instead of Webpack I have converted to using Webpack for my module bundling and NPM Scripts for my task runner. Gulp and Webpack are different in the fact that Gulp is a task runner whereas Webpack is a module bundling tool, in my example you can see I was using Browserify for module bundling within my Gulp file.
There are a few nice things that Webpack gives you such as Hot Module Reload, Webpack DevServer and really easy ways to transpile ES2015 syntax using Babel.
In addition, you can use NPM Scripts to run any task for you that is defined in your 'package.json' file, from the command line, which for me meant I could just use that for my task running rather than Gulp. I felt that I no longer needed another dependency in my projects for solely running tasks. Of course this was what I decided to use as my tooling in development, but use the tools you or your team knows and are experienced with.
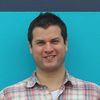
Sergio Alen
26,726 PointsInteresting, I haven't really look into it yet, but thanks Geoshepherds HB for the explanation!