Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial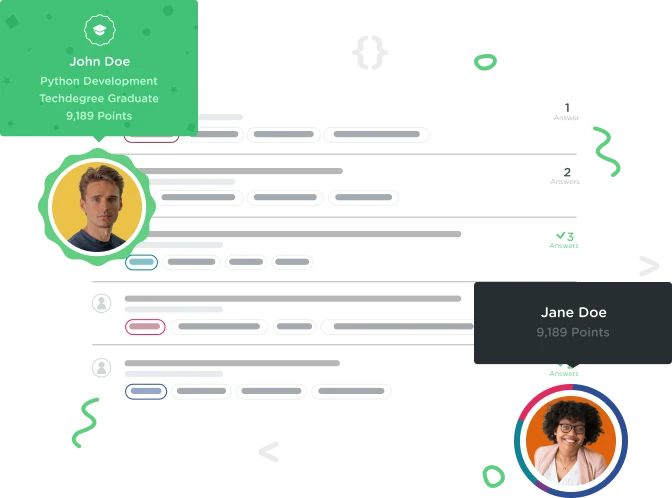
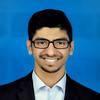
sulaiman abouabdah
5,314 Pointsusing if, !=, and or
import sys
name = input("What's your name? ")
# TODO: Ask the user by name if they understand Python while loops
# QUESTION:
answer = input('Hey {}, Do you understand Python while Loops?\n(Enter yes/no) '.format(name))
# TODO: Write a while statement that checks if the user doesn't understand while loops
# TODO: Since the user doesn't understand while loops, let's explain them.
# TODO: Ask the user again, by name, if they understand while loops.
if(answer.lower() != 'yes' or answer.lower() != 'no'):
sys.exit("Wrong input dude")
while answer.lower() == 'no':
print('Ok, {}, while loops in Python repeat as long as a certain Boolean condition is met.')
answer = input('{}, Do you understand Python while loops?\n(Enter yes/no) '.format(name))
if answer.lower() != 'yes' or answer.lower() != 'no':
sys.exit("Wrong input dude")
# TODO: Outside the while loop, congratulate the user for understanding while loops
print('That\'s great, {}, I\'m pleased that you understand while loops now. That was getting repetitive')
Whenever I enter 'yes' or 'no' It is always giving me 'wrong input dude' message instead of printing output what looping is
C:\Desktop\TeamTreehouse\Python Basics>py looping.py
What's your name? Soul
Hey Soul, Do you understand Python while Loops?
(Enter yes/no) no
Wrong input dude
C:\Desktop\TeamTreehouse\Python Basics>py
2 Answers

nimishhathalia2
6,653 PointsI can explain the logic, and where it's failing:
The way you've written the code, you want to end up with 3 scenarios:
- The user enters 'yes' then your code does something A.
- The user enters 'no' then your code does something B.
- If the user enters something else, that is, neither 'yes' nor 'no, then you print out "Wrong input dude".
Finally, because you are using the != operator, that is, a negation of 'equal to', your logical operator should also be inverted.
You want "Wrong input dude" dude to be printed out only when user provides something different than 'yes' or 'no'. But the way to write it programmatically is: Every time a user provides an input such that:
- The input is not equal to 'yes' AND
- The input is also not equal to 'no'
then do as my code says below.
In plain English, you may say a statement - my fruit is not equal to apple or to orange. But, in logical operation, you have to write the condition as follows: != 'yes' AND != 'no'
Remove "or" from your condition statements and change them to "and", whenever you have a negation mark. That should resolve your issue.
Hope this helps. Nimish.
P.S: As a matter of fact, if you were truly writing grammatically correct English, you'd write:
- My fruit equals neither the apples nor the oranges.
- Neither does my fruit equal apples, nor does it equal oranges.

nimishhathalia2
6,653 PointsNo worries! I've seen seasoned programmers miss this part, even after years of practice and experience. And what's more, it's not specific to python. Rather, this happens to almost all the languages, because we think in English or our native language, then translate it to English and then to the programming logic.
That's inherent to almost all human beings. :-)
Enjoy!
sulaiman abouabdah
5,314 Pointssulaiman abouabdah
5,314 PointsThank you so much. This helped so much. I don't know how I missed that up. Ofcourse I should have used and instead of or
Corrected code: