Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial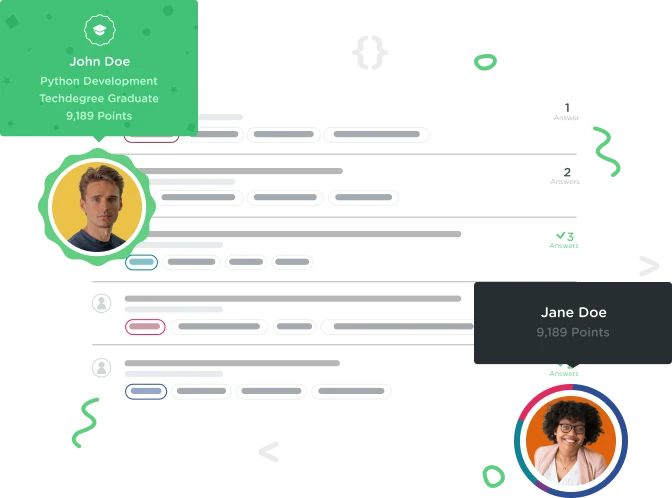

Julie Luisi
1,782 PointsUsing isinstance to keep only strings and numbers
My task is to keep only the strings and numbers from a list using isinstance and print a string of the strings and the sum of the numbers. Knowing that True has a value of 1, I need to exclude it from the sum. I ran my code with tuples, a dictionary, True and False included in a list with strings and numbers and it worked as I expected, but I bet 'Bummer: Didn't get the expected outcome.' What am I missing?
def combiner(mlist):
words = ''
nmbrs = 0
for entry in mlist.__iter__():
if isinstance(entry, str):
words += entry
elif entry is True:
nmbrs = nmbrs
elif isinstance(entry, (int, float)):
nmbrs += entry
print(words + str(nmbrs))
## ran using following list ##
#a = {'Earth': 'home}
#x = 1
#y = 2
#my_list = ['apple', True, 5.2, 'dog', 8, a, False, (x, y), (3, 5)]
#combiner(my_list)
## returns:
## appledog13.2
## as expected
3 Answers

jorgelpezguzmn
6,830 PointsUse return instead of print at the end of the function. Otherwise you are printing the value.
def combiner(mlist):
words = ''
nmbrs = 0
for entry in mlist:
if isinstance(entry, str):
words += entry
elif entry is True:
pass
elif isinstance(entry, (int, float)):
nmbrs += entry
return words + str(nmbrs)

jorgelpezguzmn
6,830 PointsIf you want to print in the console the value of words and numbers, you should use print. But the challenge is asking you to return a value, not to print it. That is the diference. If you use print, every time you call the function, you will print the result on screen, but you won't be able to asign to a variable the value the function has calculated.
If you use print, and do something like this:
new_variable = combiner(["apple", 5.2, "dog", 8])
new_variable will be empty. The function won't return anything, it will just print the results on screen.

Julie Luisi
1,782 PointsThank you for pointing that out. I missed that I needed to RETURN the value not print it. I appreciate your persistence in helping me. I completed the task. :)

jorgelpezguzmn
6,830 PointsWelcome Julie!
Julie Luisi
1,782 PointsJulie Luisi
1,782 PointsThank you for your post. I will include 'pass' in my code if entry is True. As for using return instead of print; I don't get an output with return while print gives me a string not a value because 'words' is a string and 'str(nmbrs)' makes 'nmbrs' a string as well. Still not sure why I am not succeeding at this challenge.