Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial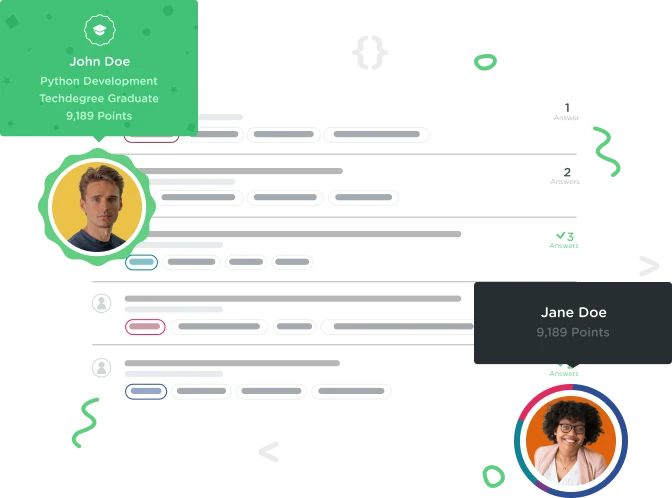

Ivan Cox
11,216 PointsUsing JavaScript to loop through JSON data and display data in particular accordion tabs
I am working on a web page where I have multiple products. Each product has a product image and an accordion with two tabs: Product features and Product information. Driving this is a JSON file which has all the Product information in it. Getting this information into the page is easy. My goal and what I am having issues with is iterating over the information in such a way that it only shows for its particular Product i.e Samsung description shows only in the second tab of the first accordion while the TCL description shows in the second tab of the second accordion, so on and so forth no matter how many items I may have.
[
{
"Prodnum": "86000SAM",
"Prodname": "Samsung 56inch LED TV",
"IsNew": "1",
"Description":[
"Using an advanced picture quality improvement algorithm",
"Samsung’s Wide Colour Enhancer Plus drastically ",
"Ultra Clear Panel",
"Resolution 1366 x 768"
]
},
{
"Prodnum": "16754TCL",
"Prodname": "TCL 42 Inch TV",
"IsNew": "0",
"Description":[
"Refresh rate 60 Hz",
"Type HDMI, USB 2.0, headphones",
"Qty 1, 3",
"Connector Type 19 pin HDMI Type A, 4 pin USB Type A, mini-phone stereo 3.5
mm"
]
}
]
my Javascript file
var url = 'https://api.myjson.com/bins/jg8rv'
var ourRequest = new XMLHttpRequest();
ourRequest.open('GET', url, true);
ourRequest.onload = function(){
var Data = JSON.parse(ourRequest.responseText)
renderInfo(Data);
}
ourRequest.send()
function renderInfo(data){
for (i = 0; i < data.length; i++)
{
if (data[i].IsNew == "1")
{
console.log("New");
}
console.log(data[i].stylenumber)
console.log(data[i].stylename)
/* Collecting Items into variables */
var list = document.getElementsByClassName('CC-Descriptions')[0];
var c = document.createElement('UL')
var item = document.createElement('li')
var descriptions = data[i].Description;
/* */
descriptions.forEach(function(descriptions){
var item = document.createElement('li')
item.innerHTML = descriptions
list.appendChild(item);
});
console.log("/*-----------------------------------------*/")
}
}
My Html
<div class="container CC-Prod-Area">
<!-- Product 1 -->
<!-- Product 1 -->
<!-- Product Area 2 -->
<div class="row mx-auto mt-5">
<div class="col color1 pt-5">
<img src="images/340-1958-0889.jpg" class="img-responsive imagespace">
</div>
<div class="col pt-3">
<div class="CC-infospace pt-5">
<div class="CC-New"></div> <div class="CC-Style-Info"></div>
<div id="accordion" class="accordion">
<div class="card">
<div class="card-header collapsed" data-toggle="collapse" data-parent="#accordion1" href="#collapseOne">
<a class="card-title">
Colors
</a>
</div>
<div id="collapseOne" class="card-block collapse show">
<ul class="CC-Descriptions">
Information
</ul>
</div>
<div class="card-header collapsed" data-toggle="collapse" data-parent="#accordion2" href="#collapseTwo">
<a class="card-title">
Product Information
</a>
</div>
<div id="collapseTwo" class="card-block collapse">
<ul class="CC-Descriptions">
<div class="CC-Prodname">Product Descriptions</div>
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="row mx-auto mt-5">
<div class="col color1 pt-5">
<img src="images/340-1958-0889.jpg" class="img-responsive imagespace">
</div>
<div class="col pt-3">
<div class="CC-infospace pt-5">
<div class="CC-New"></div> <div class="CC-Style-Info"></div>
<div id="accordion" class="accordion">
<div class="card">
<div class="card-header collapsed" data-toggle="collapse" data-parent="#accordion3" href="#collapseThree">
<a class="card-title">
Colors
</a>
</div>
<div id="collapseThree" class="card-block collapse show">
<ul class="CC-Descriptions">
Information
</ul>
</div>
<div class="card-header collapsed" data-toggle="collapse" data-parent="#accordion3" href="#collapseFour">
<a class="card-title">
Product Information
</a>
</div>
<div id="collapseFour" class="card-block collapse">
<ul class="CC-Descriptions">
<div class="CC-size"></div>
<div class="CC-Prodname">Product Descriptions</div>
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>