Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial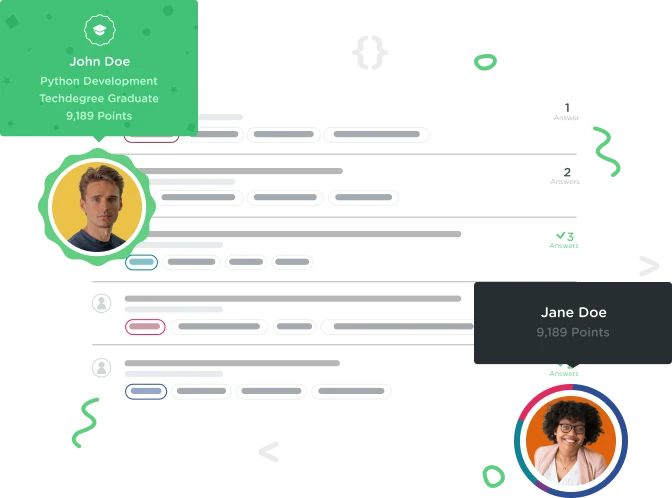

Alexander Karan
Courses Plus Student 15,220 PointsUsing Layout Anchors
So am I'm doing the below code challenge and I'm sure i have the code right but it keeps telling me I'm wrong. Any thoughts people?
Question: In the editor, you've been provided with a view controller subclass that contains two instances of UIView as stored properties. The work to set up blueView has already been done; you need to set up greenView.
To set up the green view add the following constraints: Pin to the bottom of blueView with 8 point spacing Pin to left and right margins with 8 point spacing Add height constraint of 75 points.
For this exercise you may use the verbose NSLayoutConstraint APIs or layout anchors to create constraints.
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: blueView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .Width, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .Left, relatedBy: .Equal, toItem: view, attribute: .LeftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Top, relatedBy: .Equal, toItem: self.topLayoutGuide, attribute: .Bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Right, relatedBy: .Equal, toItem: view, attribute: .RightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
// I added this bit below
NSLayoutConstraint.activateConstraints([
greenView.heightAnchor.constraintEqualToConstant(75.0),
greenView.topAnchor.constraintEqualToAnchor(blueView.bottomAnchor, constant: -8.0),
greenView.rightAnchor.constraintEqualToAnchor(view.layoutMarginsGuide.rightAnchor, constant: -8.0),
greenView.leftAnchor.constraintEqualToAnchor(view.layoutMarginsGuide.leftAnchor, constant: 8.0)
])
}
}
}
5 Answers
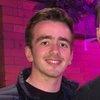
Stephen McMillan
iOS Development with Swift Techdegree Graduate 33,994 PointsHi,
Looking at this Apple documentation for NSLayoutGuide i was able to pass this challenge by modifying your code to:
greenView.heightAnchor.constraintEqualToConstant(75.0).active = true
greenView.topAnchor.constraintEqualToAnchor(blueView.bottomAnchor, constant: 8.0).active = true // You also had this as -8 and it should be 8.
greenView.rightAnchor.constraintEqualToAnchor(view.rightAnchor, constant: -8.0).active = true
greenView.leftAnchor.constraintEqualToAnchor(view.leftAnchor, constant: 8.0).active = true
Also, what I've put above doesn't need to be in NSLayoutGuide.activateConstaints.
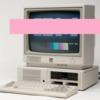
kols
27,007 PointsUnfortunately, I couldn't get either of these to work ^^. Probably just been so long since originally posted; also I noticed that the original code looks like it's may have changed slightly from what is shown above to what's in the current challenge.
I chose to do the verbose NSLayoutConstraint APIs version, and this is what worked successfully for me:
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
NSLayoutConstraint(item: blueView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: self.topLayoutGuide, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
NSLayoutConstraint.activate([
NSLayoutConstraint(item: greenView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 75.0),
NSLayoutConstraint(item: greenView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .top, relatedBy: .equal, toItem: blueView, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])
}
}
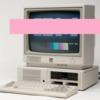
kols
27,007 Points^^ In response to my previous post, I've gone back and reworked some of these challenges now. Here is an alternate answer using anchors (both of these answers still work as of this time - Dec 2017 - in Swift 4):
Here's the link to Apple's documentation re: Anchors: Apple | NSLayoutAnchor for anyone looking for add'l info.
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
NSLayoutConstraint(item: blueView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: self.topLayoutGuide, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
greenView.topAnchor.constraint(equalTo: blueView.bottomAnchor, constant: 8.0).isActive = true
greenView.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 8.0).isActive = true
greenView.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: -8.0).isActive = true
greenView.heightAnchor.constraint(equalToConstant: 75.0).isActive = true
}
}

Matthew Ingram
Courses Plus Student 4,718 PointsNSLayoutConstraint.activateConstraints([ greenView.heightAnchor.constraintEqualToConstant(75.0), greenView.topAnchor.constraintEqualToAnchor(blueView.bottomAnchor, constant: -8.0), greenView.rightAnchor.constraintEqualToAnchor(view.rightAnchor, constant: -8.0), greenView.leftAnchor.constraintEqualToAnchor(view.leftAnchor, constant: 8.0) ])

Angus Muller
iOS Development Techdegree Graduate 12,473 PointsDid not help I was working in Swift 3. I wanted to use anchor but gave up and used the below that worked for me.
NSLayoutConstraint.activate([
NSLayoutConstraint(item: greenView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 75.0),
NSLayoutConstraint(item: greenView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .top, relatedBy: .equal, toItem: blueView, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])

Jos Feseha
4,011 PointsNSLayoutConstraint.activate([
NSLayoutConstraint(item: greenView, attribute: .bottom, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .height, relatedBy: .equal, toItem: self.topLayoutGuide, attribute: .bottom, multiplier: 1.0, constant: 75.0),
NSLayoutConstraint(item: greenView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])