Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial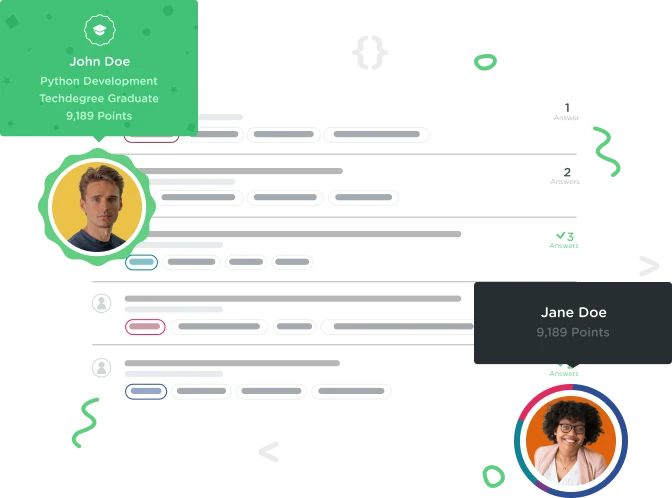

Aaron Figueroa
2,860 Pointsusing logical operator with this code is saying "unexpected end of input, expecting keyword_end. why?
I feel like the code is rock solid but no matter how I change it up, it doesn't seem to work. why?
def check_speed(car_speed)
car_speed = gets.chomp.to_i
if (car_speed >= 40) && (car_speed <= 50)
return "safe"
end
7 Answers
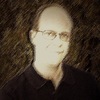
Jason Anders
Treehouse Moderator 145,858 PointsHey Aaron,
Chi-Shiang Wang is right about needing an end
for your if
statement, but you also added a line that was not asked for by the challenge (challenges are very strict).
If you add the end
statement and delete the line car_speed = gets.chomp.to_i
, you will be good to go.
Completed code will look like this:
def check_speed(car_speed)
if (car_speed >= 40) && (car_speed <= 50)
return "safe"
end
end
Hope that helps and Keep Coding! :)
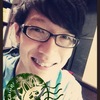
Chi-Shiang Wang
3,471 PointsYou need add a "end" tag when your if-statement is finish.

Aaron Figueroa
2,860 PointsHey Chi- Shiang Wang! Thank you for answering the question but Im still getting an error. I was wondering if I was still doing something wrong. i just put an end tag after my if statement and it just says 'bummer' and try again. Is the code solid?
This is what it said: set the return value of the "check_speed" method to the string "safe" depending on the following conditions: the speed passed in as an argument is at least 40 the speed passed in as an argument is less than or equal to 50
you should use the && logical operator to accomplish this task.
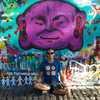
Andrew Stelmach
12,583 PointsHi Aaaron, have you completed this challenge yet? I tried it and it passes with what you say you did in your latest comment. Maybe you've done a typo? I wouldn't usually recommend just giving someone an answer but, since you've tried a few things already:
def check_speed(car_speed)
if car_speed >= 40 && car_speed <= 50
"safe"
end
end
Notice lack of 'return'. Return is not needed for this method. Return is used when you want to return a value and exit the method immediately without running any code that is after that statement in the method. Since, if the condition in our 'if' statement is not met, we're happy for the rest of the method to run and return 'nil', we don't need a 'return' statement in this method.
Also, a return value of a method is equal to its last evaluation, so we can just state "safe" and that will be the return value of the method (if the if condition is met). No need for 'return'.
Also, careful with your indentation - the first line of your method in your original post needs to be indented because it is nested within the method.
In your original answer it's worth noting the misuse of gets.chomp.to_i; to use the method, the user must call the method with an argument, and for the sake of the tutorial, we can assume the user knows to pass in a number. So, the method will be called as something like:
check_speed(42)
Therefore, we will already have a value for 'car_speed' - no need to ask the user to input it after the method has been called.
If the method is called without passing in something as an argument, a Ruby error will be raised and the method will not run.
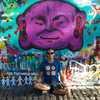
Andrew Stelmach
12,583 PointsPeople often prefer one-line syntax for conditionals:
def check_speed car_speed
"safe" if car_speed >= 40 && car_speed <= 50
end
Note that no extra 'end' is needed with this syntax. This syntax is not always best - sometimes the long-hand method is better for readability. In this particular case, personally I think this syntax is better, but it's personal preference.

Jeremy Hurtt
2,940 PointsHow is this wrong -
def check_speed(car_speed)
if (car_speed >= 40) && (car_speed <= 50)
puts "safe"
end
end
?
This is the third or fourth time I've been entering code in exactly how I was told to, and the system gives absolutely no clue why it isn't being accepted. Can anyone please tell me what is wrong with this? This is EASILY my biggest pet peeve with treehouse so far, the total lack of explanation when it tells you you're wrong...that really doesn't work in tandem with a system that apparently demands a strict use of syntax.
I have copied and pasted every answer provided in this thread, and none worked.
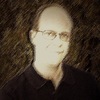
Jason Anders
Treehouse Moderator 145,858 PointsYou are missing the else
statement that the challenge asks for. The challenge needs a completed if/else
statement.

Jeremy Hurtt
2,940 PointsYeah, I have done it with the else statement, without it, I've tried it 100 different ways. What I posted was only the simplest, most-stripped down, most recent version. For instance,
def check_speed(car_speed)
if (car_speed >= 40) && (car_speed <= 50)
puts "safe"
else
puts "unsafe"
end
end
Does not work.
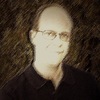
Jason Anders
Treehouse Moderator 145,858 PointsOkay Jeremy,
Your code is completely correct, except the challenge is asking you to return "safe or "unsafe", and you are using puts
instead of return
.
Just change those and it will pass. :)

Jeremy Hurtt
2,940 PointsOk, it's working now. I tried about a dozen different versions with "return" earlier and none of them worked, but who the hell knows. Thank you.
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 Pointsreturn
not necessary, but could be argued it's more readable. Personally, I'd leave it out, but it comes down to personal preference.