Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial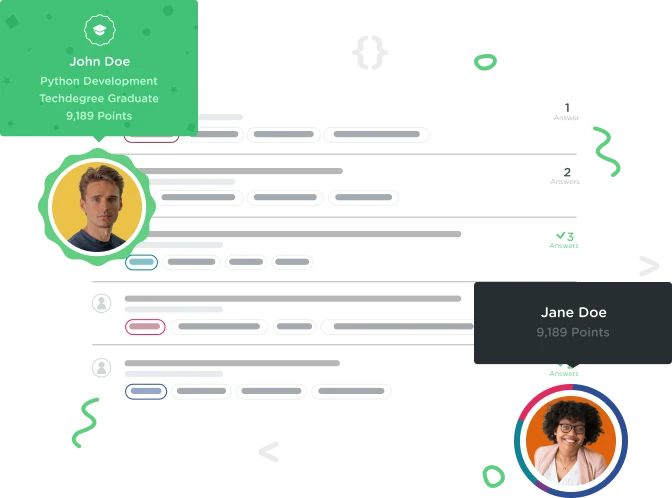

James Croxford
12,723 PointsUsing loops for JavsScript Arrays-why can JS function parameters/arguments be declared without specifying the data type?
I've previously done a little of Java. So coming from that language, I'm used to seeing argument lists for methods/functions being comprised of pairs of data types and associated variable names. The method therefore knows what type of variable to expect.
Here in JS, there doesn't yet seem to be any requirement to specify the data type for any arguments named within the declaration of a function. What is the technical reason for this? Does this not create issues during development if the wrong data type is passed to the function? Are there ways around this?
For example, my code below. I've commented out the array of songs from the class, and created two alternative variables to experiment with:
//let playList = [
// 'I Did It My Way',
// 'Respect',
// 'Imagine',
// 'Born to Run',
// 'Louie Louie',
// 'Maybellene'
//];
//alternative value no. 1:
let playList = 6;
//alternative value no.2:
//let playList = '6';
function print(message) {
document.write(message);
}
function printList( list ) {
let listHTML = '<ol>';
for (let i = 0; i < list.length; i += 1) {
listHTML += `<li> ${list[i]} </li>`;
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
Of course the array from the class prints to the screen as intended. If I use the first alternative value for the variable playList (6), which is an int, no list prints to the page (maybe because I haven't used parseInt?). The console on my browser doesn't show an error code. If I use the second alternative, the string of '6', then that prints to the screen as the only item in the ordered list.
Surely by not setting an expected type to the parameter in the function printList, this opens up the possibility that the wrong data type could be passed to the function and require manually checking the code/output on the page? Here it isn't an issue as the program is tiny, but when trying to debug a large program this would be a huge hassle right?
1 Answer
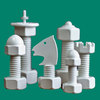
Steven Parker
231,248 PointsJavaScript is what is known as a loosely typed or a dynamic language. This gives it greater flexibility, but as you point out it also creates possibilities for errors that might be detected by the compiler in a strictly-typed compiled language.
There exists a superset language called "TypeScript" that includes type checking as part of an added transcompilation step. For more information, see this Wikipedia page on TypeScript.
James Croxford
12,723 PointsJames Croxford
12,723 PointsThanks for the response! TypeScript sounds intriguing!
Steven Parker
231,248 PointsSteven Parker
231,248 PointsThere's also a Getting Started with TypeScript workshop here on Treehouse.