Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial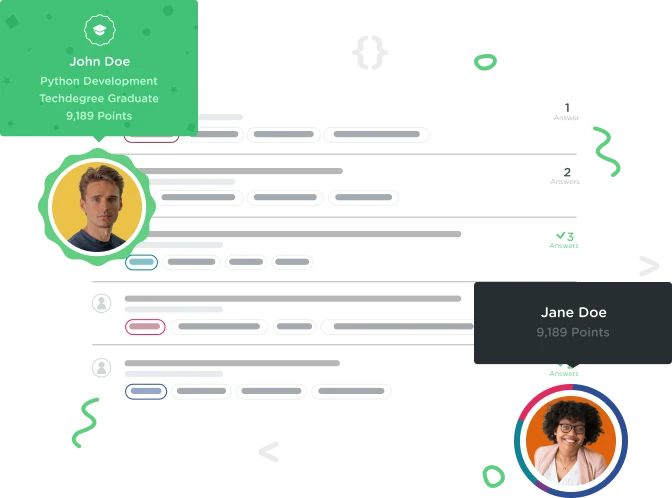
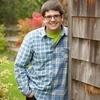
Sam Dale
6,136 PointsUsing Multiple "== statements" vs "or statements"
Hi there. This is a simple question, but Python has the weird and unique ability to do crazy stuff like 3 < x < 5 checks. So Kenneth has a line:
if monster == door or monster == start or start == door:
The line that follows could do the same thing in a more concise way:
if monster == door == start:
I know functionality-wise it doesn't work and this is an oddity that applies only to Python, but is the second way more Pythonic? Or is the first one more clear? Thanks!
2 Answers
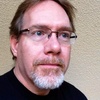
Chris Freeman
Treehouse Moderator 68,457 PointsYour first condition will stop evaluating at the first matching pair since the other matches can not make the result any more True:
monster == door or monster == start or start == door
The second condition
monster == door == start
This is equivalent to
# monster == door and monster == start and start == door # not correct
monster == door and door == start # correction by pointed out by @[Jason Anello](https://teamtreehouse.com/jasonanello)
That is, it's True only if they all match. But if you reverse the comparison, it will be True only if they all differ:
>>> start = 2
>>> door = 4
>>> monster = 6
>>> start, door, monster
(2, 4, 6)
>>> start == door == monster
False
>>> start != door != monster
True
EDIT: as pointed out by Jason Anello this is equivalent to
start != door != monster
# is equivalent to
start != door and door != monster
As mentioned further down, adding an additional round-robin case fixes it:
start != door != monster != start
# is equivalent to
start != door and door != monster and monster != start
Note: This is a only a syntax curiosity. The Pythonic way is to spell it out with the long form.
start != door and door != monster and monster != start
# OR
start == door and door == monster and monster == start
depending on your goals.
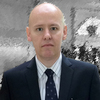
Nathan Tallack
22,160 PointsJust remember, the moment it gets match it will stop evaluating.
So for your first one it will never check your third conditional if the first or second match.
For your second one it will only work if all three match.
Sam Dale
6,136 PointsSam Dale
6,136 PointsRight. I totally overlooked that. Thanks!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHey Chris,
I think
monster == door == start
would be equivalent to
monster == door and door == start
monster and start wouldn't be compared against each other.
Also, your final example where you reverse the comparisons will also be True if start and monster are equal but different from door.
It's equivalent to
start != door and door != monster
This doesn't restrict start and monster from being equal to each other.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsAck! You're right! Thanks Jason. I was too quick with insufficient tests. Adding one more round-robin test fixes it:
I'll amend my answer above