Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial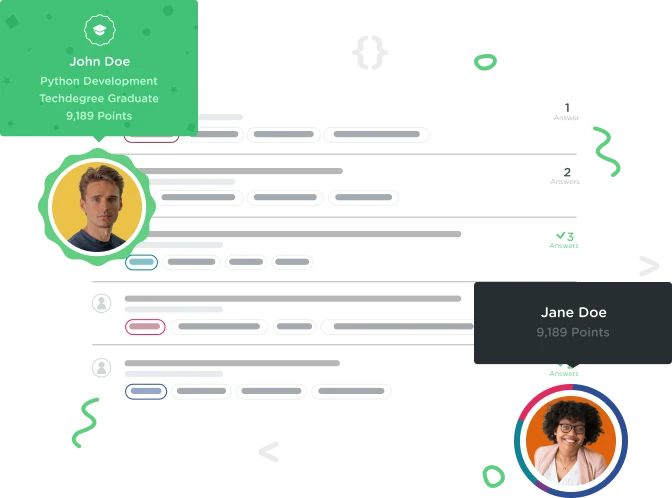

furkan
11,733 PointsUsing objects, functions, and arrays. Creating a simple game module
Here is a basic example of question and answer game module, making use of several important aspects of JavaScript. A great example for those still learning JavaScript (including myself). I have created the game module below, combining some very important characteristics of JavaScript: Object Literals, Arrays, Functions, Switch Statements.
I have provided this module with some helpful comments (hopefully) to get you to understand what is happening in the code. Feel free to copy and paste into your console to enjoy the simple 4 question and answer game:
// Create a game object with the name gameObject
var gameObject = {
player: undefined, // Currently undefined, but will update during the game
points: 0, // This will keep track of the player score and updates during game
/* The questions key (below) inside this object, holds an array with the questions to ask the player */
questions: ['What is the capital of England?', 'What is the capital of Turkey?',
'What is the capital of USA?', 'What is the capital of Japan?'],
answers: 0, // This will keep track of the player score
/*The checkWin function will run at the end of the game to check the score and see if the player has won the game or not, by getting 3 or more correct answers */
checkWin: function() {
if (this.answers >= 3){ // The condition checks to see if 3 or more correct answers
return console.log('You have won the game!'); // Returns true if 3 or more
} else{
console.log('You lose. Better luck next time!'); // Otherwise returns false
}
}
}
/* startGame function below makes use of all the keys in the object literal shown above to help create the game */
function startGame(){ // Creates a function with the name startGame
var getName = (prompt('What is your name?') );
getName; // First get the player name and store in getName variable
/* Once the player name is inserted, update the player key in the gameObject shown below*/
gameObject.player = getName;
var level = 1; // Sets the context to begin the switch statements
switch(level){
/* All the cases below use a prompt function with a .toLowerCase() to prevent any confounding errors from player typing answer. The .toLowerCase() returns all answers as lower case, thus, keeping it consistent in the code*/
case (1): var questionOne = prompt(gameObject.questions[0]).toLowerCase();
if(questionOne === 'london'){ /* note how the answers are all lower case because we used the .toLowerCase() for the prompt where users type their answers. Each time the player gets a correct answer, we update the gameObject points and answers witht eh following codes shown below */
gameObject.points += 100;
gameObject.answers += 1;
}
case (2): var questionTwo = prompt(gameObject.questions[1]).toLowerCase();
if(questionTwo === 'ankara'){
gameObject.points += 100;
gameObject.answers += 1;
}
case (3): var questionThree = prompt(gameObject.questions[2]).toLowerCase();
if(questionThree === 'washington' || questionThree === 'washington d.c.'){
gameObject.points += 100;
gameObject.answers += 1;
}
case (4): var questionFour = prompt(gameObject.questions[3]).toLowerCase();
if(questionFour === 'tokyo'){
gameObject.points += 100;
gameObject.answers += 1;
}
/* Also, note how break; statements are not used in each case shown above. If break; statement were to be, for example, for case(1), rest of the following cases would be skipped and the game would not work properly. */
}
/* In the code below, we are storing the players name, their points and correct answers given in the game and then calling that in console.log(). */
var totalPoints = getName + ', your total points are: ' + gameObject.points + ', and total correct answers: ' + gameObject.answers + '/4';
console.log(totalPoints);
gameObject.checkWin(); // Final code checks to see if the player has won.
}
/*Code below calls the startGame function to begin the game. If we skip this code, the game would not begin. Thus, this is why programmers say that functions are callable, because we can just call a function to run the codes only when we want it to. */
startGame();
I hope the code and explanation above has helped new learners a little bit about the uses of objects, functions, arrays and switch statements.
furkan
11,733 Pointsfurkan
11,733 PointsBelow is the same code but without the comments: