Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial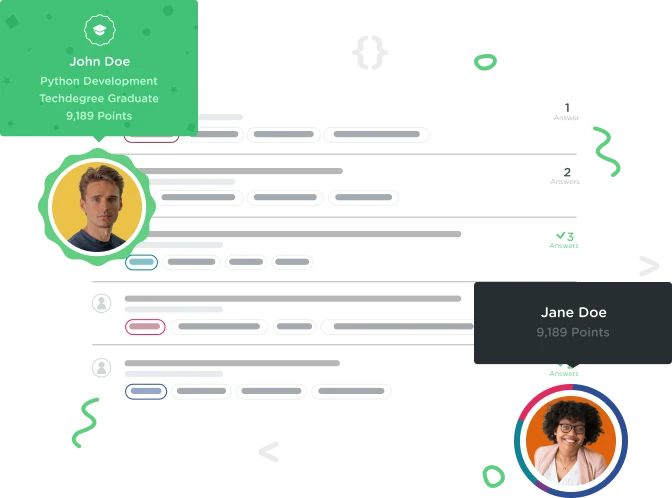

Andrew Goninon
14,925 PointsUsing OR in an IF statement
Hi,
I'm trying to make a shortcut for "reverse" and "uppercase" by allowing the user to type "r" and "u" respectively. What would be the correct way of implementing this because the code below does not seem to work.
Thanks!
print "Enter name: "
name = gets.chomp.capitalize
if name == "Andrew"
puts "That's my name, too!"
else
puts "Hi #{name}!"
end
print "Modify your name (reverse or uppercase): "
response = gets.chomp.downcase
if response == ("reverse" || "r")
puts "Your name in reverse is: #{name.reverse}"
elsif response == ("uppercase" || "u")
puts "YOUR NAME IS: #{name.upcase}!"
elsif response == "both"
puts "Your name in reverse and uppercase is: #{name.reverse.upcase}!"
else
puts "That wasn't one of the options, learn to read!"
end
1 Answer

Andrew Goninon
14,925 PointsOkay, so it touches on this a few lessons later. See the code below for the answer.
Using IF
print "Enter name: "
name = gets.chomp.capitalize
if name == "Andrew"
puts "That's my name, too!"
else
puts "Hi #{name}!"
end
print "Modify your name (reverse or uppercase): "
response = gets.chomp.downcase
if (response == "reverse") || (response == "r")
puts "Your name in reverse is: #{name.reverse}"
elsif (response == "uppercase") || (response == "u")
puts "YOUR NAME IS: #{name.upcase}!"
elsif (response == "both")
puts "Your name in reverse and uppercase is: #{name.reverse.upcase}!"
else
puts "That wasn't one of the options, learn to read!"
end
Using CASE
print "Enter name: "
name = gets.chomp.capitalize
if name == "Andrew"
puts "That's my name, too!"
else
puts "Hi #{name}!"
end
print "Modify your name (reverse or uppercase): "
response = gets.chomp.downcase
case response
when "reverse", "r"
puts "Your name in reverse is: #{name.reverse}"
when "uppercase", "u"
puts "YOUR NAME IS: #{name.upcase}!"
when "both"
puts "Your name in reverse and uppercase is: #{name.reverse.upcase}!"
else
puts "That wasn't one of the options, learn to read!"
end