Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial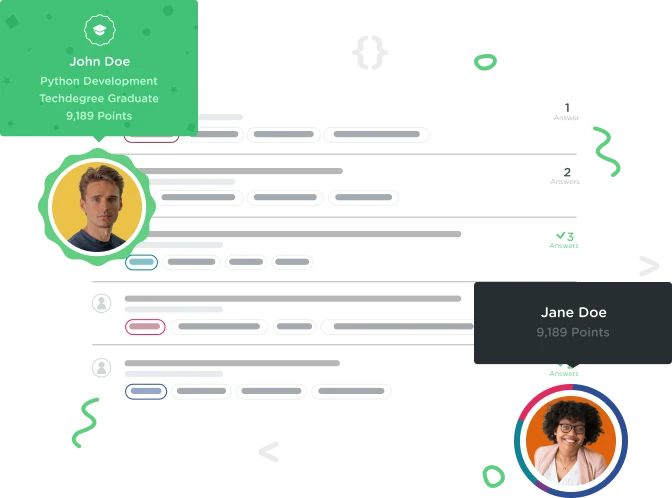
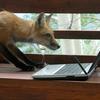
Daniel McNeil
Full Stack JavaScript Techdegree Graduate 26,471 PointsUsing Pug with Angular 2
I am taking the Angular Basics Course (Angular 2) and I would like to use Pug for my component templates. I followed the directions here:
https://github.com/AngularClass/angular2-webpack-starter/wiki/How-to-add-.pug-support
I added pug and pug-html-loader to my dev dependencies:
{
"name": "photoblog",
"scripts": {
"postinstall": "npm run build",
"serve": "webpack-dev-server --config config/develop.config.js --inline --progress --port 8080",
"build": "webpack"
},
"dependencies": {
"@angular/common": "2.4.2",
"@angular/compiler": "2.4.2",
"@angular/core": "2.4.2",
"@angular/platform-browser": "2.4.2",
"@angular/platform-browser-dynamic": "2.4.2",
"core-js": "2.4.1",
"ie-shim": "0.1.0",
"rxjs": "5.0.3",
"zone.js": "0.7.4"
},
"devDependencies": {
"@types/core-js": "0.9.35",
"@types/node": "6.0.59",
"angular2-template-loader": "0.6.0",
"awesome-typescript-loader": "3.0.0-beta.18",
"css-loader": "0.26.1",
"extract-text-webpack-plugin": "1.0.1",
"file-loader": "0.9.0",
"html-loader": "0.4.4",
"html-webpack-plugin": "2.26.0",
"json-loader": "0.5.4",
"pug": "^2.0.0-beta11",
"pug-html-loader": "^1.1.1",
"raw-loader": "0.5.1",
"script-loader": "0.7.0",
"source-map-loader": "0.1.5",
"style-loader": "0.13.1",
"to-string-loader": "1.1.5",
"ts-loader": "1.3.3",
"tslint": "4.3.1",
"tslint-loader": "3.3.0",
"typescript": "2.1.4",
"uglify": "0.1.5",
"uglify-loader": "1.4.0",
"webpack": "1.14.0",
"webpack-dev-server": "1.16.2",
"webpack-merge": "2.3.1"
}
}
I added pug-html-loader to the module loader in config/common.config.js:
const path = require('path');
const webpack = require('webpack');
const HtmlWebpackPlugin = require('html-webpack-plugin');
const ExtractTextPlugin = require('extract-text-webpack-plugin');
const extractCSS = new ExtractTextPlugin('[name].css');
const ROOT = path.resolve(__dirname, '..');
function root(pathParts) {
if (typeof pathParts === 'string') {
pathParts = pathParts.split('/');
}
return path.join.apply(path, [ROOT, ...pathParts]);
}
const context = root('src');
module.exports = {
context,
entry: {
polyfills: './polyfills.ts',
vendor: './vendor.ts',
app: './main.ts'
},
output: {
path: root('wwwroot'),
publicPath: '/',
filename: '[name].[hash].js',
chunkFilename: '[id].[hash].chunk.js'
},
resolve: {
extensions: ['', '.ts', '.js']
},
module: {
loaders: [
{
test: /\.ts$/,
loaders: ['awesome-typescript-loader?configFileName=' + root('src/tsconfig.json'), 'angular2-template-loader']
},
{
test: /\.html$/,
loader: 'html'
},
{
test: /\.(png|jpe?g|gif|svg|woff|woff2|ttf|eot|ico)$/,
loader: 'file?name=/assets/[name].[hash].[ext]'
},
{
test: /\.css$/,
exclude: root('src'),
loader: ExtractTextPlugin.extract('style', 'css?sourceMap')
},
{
test: /\.css$/,
include: root('src'),
loader: 'raw'
},
{
test: /\.css$/,
include: root('src/styles'),
loader: extractCSS.extract(['css'])
},
{
test: /\.pug$/,
loader: 'pug-html-loader'
}
]
},
plugins: [
new webpack.optimize.CommonsChunkPlugin({
name: ['app', 'vendor', 'polyfills']
}),
new HtmlWebpackPlugin({
template: 'index.html'
}),
extractCSS
]
};
edited my app.component.ts to call the pug template:
// import component module
import {Component} from '@angular/core';
// component decorator - mark a class as a component and set configuration metadata
@Component({
// custom html tag
selector: 'app-root',
// select view
templateUrl: 'app.component.pug',
// stylesheet
styleUrls: ['app.component.css']
})
// name of the component
export class AppComponent {
emoji: string[] = this.emoji = ['?', '?', '?', '?'];
activeEmoji: string;
changeEmoji() {
this.activeEmoji = this.emoji[Math.floor(Math.random() * this.emoji.length)];
}
}
and created an app.component.pug file:
h2 Hello World! {{emoji}}
When run 'npm run serve' I get an error from webpack:
ERROR in ./src/app/app.component.pug
Module parse failed: C:\Users\c330716.EXECUTIVE\Documents\Code\photo_blog\node_m
odules\pug-html-loader\lib\index.js!C:\Users\c330716.EXECUTIVE\Documents\Code\ph
oto_blog\src\app\app.component.pug Unexpected token (1:0)
You may need an appropriate loader to handle this file type.
Are there compatibility issues between webpack and pug? Does it have something to do with the versions of webpack and pug that I'm using?