Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial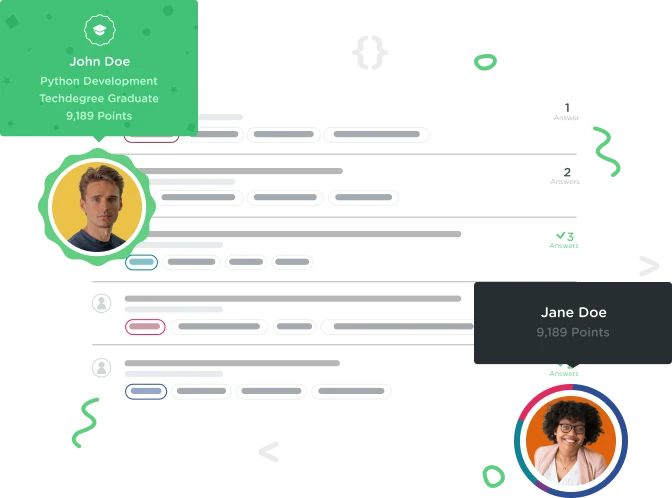

Chris Gauthier
Courses Plus Student 6,434 PointsUsing Queues
Hello, can somebody have a look at this? While, I think I understand the concept of queueing now, especially the add to queue and poll queues, but I suspect my logic and implementation is suspect.
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while (mSupportReps.poll == null) {
playHoldMusic();
}
acceptCustomer(customer);
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHi Chris
Yes, you have begin to understand the concept behind Java Queue implementations. However, there are some small details about the project you have been missed out. Let's start from TODO #1:
The main key here is the hint: you need to make while assignment check which means assign the csr while checking if the value returned null or not. Thus your code should look like this:
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while ((csr=mSupportReps.poll()) == null) {
playHoldMusic();
}
As you can see I assigned the csr by using mSupportReps.poll() Queue method and in the same time checking if csr is null or not. If it still returns null playHoldMusic.
Next TODO#2: Since we already assign csr as CustomerSupportRep Object from mSupportReps Queue we can use the assist method directly to make the newly assigned csr to assists customer. The code should look like this:
//acceptCustomer(customer);<-- DELETE THIS
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.assist(customer);
For the TODO#3 you have done just fine. As I said you started to get a hold on the Queue implementation concept. You just need to fix your code on the TODO#1 and TODO#2 and you will be just fine.
I hope this can help. Happy coding

Chris Gauthier
Courses Plus Student 6,434 Pointsyeah, that did the trick!