Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial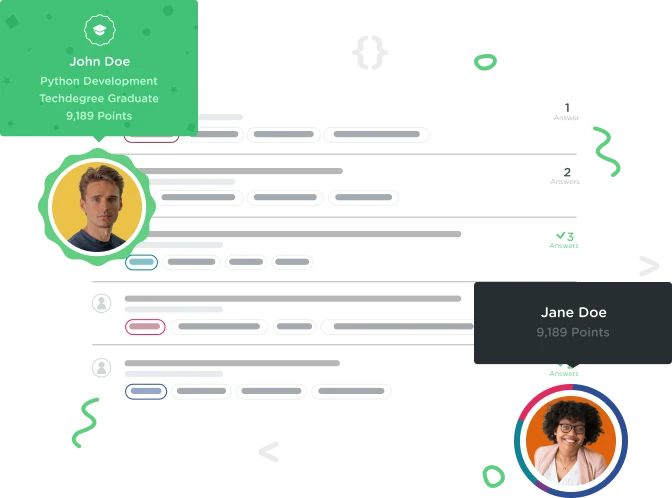

Marquis Briggs
8,967 PointsUsing reduce, return the total phone numbers with a 503 area code. Store the total in the variable numberOf503.
Not sure what the problem is
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberof503 = phoneNumbersreduce(count, phoneNumber) =>
(phoneNumber.includes('(503)')){
count +=1;
}
return count;
},0);
1 Answer
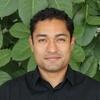
Juan Luna Ramirez
9,038 Pointsconst phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// 1. forgot the period between phoneNumbers and reduce
// 2. take care when passing in a function to another function.
// You are missing a parentheses for the arguments. Also the
// curly braces for the body of the reducer function.
numberof503 = phoneNumbers.reduce((count, phoneNumber) => {
// 4. use if statement
if (phoneNumber.includes('(503)')){
count +=1;
}
return count;
} ,0);
I often mess up the syntax for the reducer function so usually I will do this:
-
setup an empty reducer function as the first argument and the initial value as the second argument of
reduce
numberOf503 = phoneNumbers.reduce(() => {}, 0)
-
add the accumulator and item parameters to the reducer function
numberOf503 = phoneNumbers.reduce((count, phoneNumber) => {}, 0)
-
break up at the body and begin writing the body of the reducer function
numberOf503 = phoneNumbers.reduce((count, phoneNumber) => { // reducer function logic here }, 0)