Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial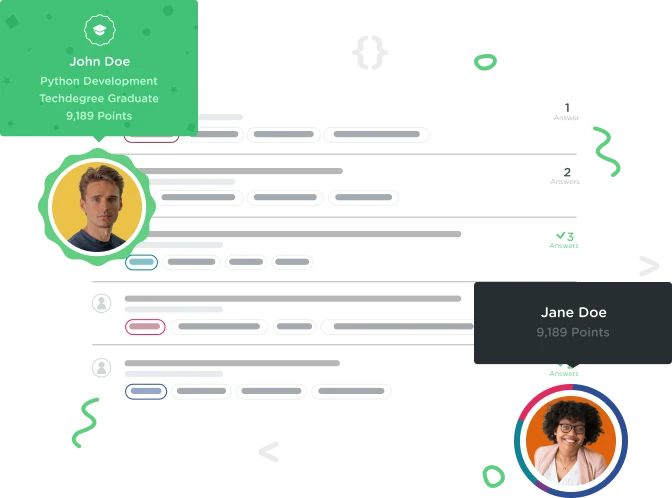
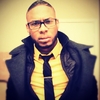
Kirk Lewis
1,486 PointsUsing same property names within different parts of blocks of code is confusing me.
So I've noticed as I have been going through this course that there are times when the same keywords are used in a block of code. The keywords are typically parameters and / or variables of some sort and what makes it confusing is that when that certain keyword is referenced I cannot quickly understand if the instructor is referencing the variable, or the parameter or whatever it may be.
Here's an example: Below we have a class. In this class it looks like we have two variable aspects that could, or could not be initialized right then. In this particular example the price was initialized but still in the init the price is initialized again? Why?
Additionally, I am not understanding what the self.price and self.title is referring to because it's typed so many times in this one block of code. I can't immediately understand if self.title = title is referring to what is being passed when the class is called? If so, how is that initializing if it's not defined until this value is passed in the parameters when the class is being called?
If you look at the code and see how many times the word title and price are found you will understand the core of my confusion. I cannot tell which is being reference to what... the hierarchy, if any, and what is referencing what.
I am looking for someone to help me understand this.
class Product2{
var title: String
var price: Double = 0.0
init(title: String, price: Double){
self.title = title
self.price = price
}
}
let quadcopter2 = Product2 (title: "Quadcopter", price: 499.99)
I understand that it works. And I can copy the model and move on... but by doing so I would not be learning. Someone please help me and I thank you for taking the time out in advance!
-Kirk II
1 Answer
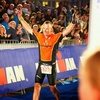
Steve Hunter
57,712 PointsHi Kirk,
You have a class called Product. This is the template for making many instances of Product with each being defined by their price and title.
The initialiser method is called when an instance of the class is requested. Its job is to ensure that the instance/object created is created properly, to your specification - you'll have seen default initialisers and convenience ones, or you soon will.
In this example, the initialiser takes two parameters. They've been called the same as the class instance varialbles but don't need to be:
init(name: String, cost: Double){
self.title = name
self.price = cost
}
That would work just the same.
The self
prefix is designed to make it clear which variable you are referencing. Within the original initialiser,
init(title: String, price: Double){
self.title = title
self.price = price
}
The self
means that you are setting the instance's own variable; the member variable. The self
is not needed. If you use Xcode to define which version of title
and price
you want (the drop-downs give you the option) you can omit the self
entirely but that lacks clarity of code.
Why is the Double initialised twice? Not sure, this may be due to the order the course progressed in. It might be a throwback to the class before the init
method existed. It isn't necessary to do that.
So, the key thing here is to contrast a class and a function. You are talking about "calling" the class. That's not what happening here. You are creating an instance of the class. That creates a stand-alone object generated by the template set out in the "master copy" of the class.
The parameters pass in as the object is created are those parameters required to create the object - after that's done you have a product with a name and a price that is only related to the class code by duplication. It isn't like a function that is called repeatedly and the same code executes over and over. If you only create one instance, the class code will run once. But you can perform many tasks with that one object, using its functionality multiple times. (Not this class - it does nothing!!).
I hope that makes some sense!
Steve.
Kirk Lewis
1,486 PointsKirk Lewis
1,486 PointsIt all makes sense and thank you so much for the well-written explanation Steve!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThanks Kirk - I'm glad I could help.
Feel free to tag me on here if you need some help in the future, or tweet me @OnlySteveH; I'll hear you!
Steve.