Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial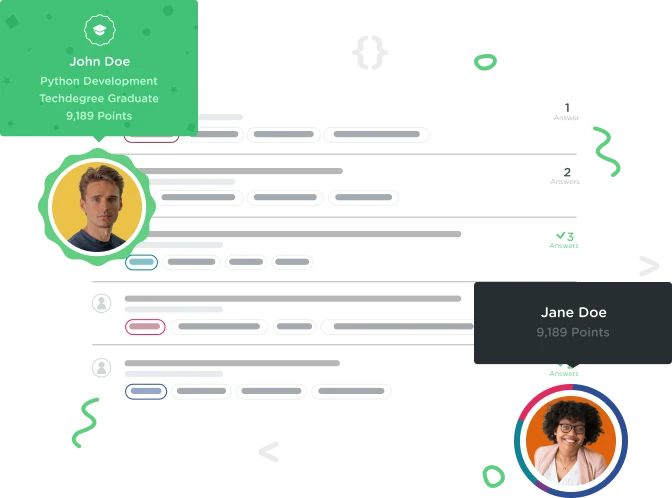
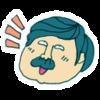
Fernando Cordeiro
34,597 PointsUsing SCSS in a Django project
Hi, is there an official or most recommended way to compile SCSS in a Django project?
I was surprised it didn't ship with one out of the box, considering it's a batteries-included framework, and thought I was missing something.
On github I found django-libsass (last updated 2 years ago) and django-static-precompiler (7 months ago), both with a surprisingly low number or stars, which made me wary.
What do you guys use?
1 Answer
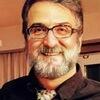
Kyle Hartigan
16,967 PointsHi Fernando,
As Django is a backend web framework, and SCSS is a front-end style sheet language they are not very connected. This is why it doesn't work "out of the box". In order to compile SCSS you need a task runner. The two most popular task runners are Gulp and Grunt. I personally prefer Gulp.
Here is a course on TreeHouse: https://teamtreehouse.com/library/gulp-basics
Fernando Cordeiro
34,597 PointsFernando Cordeiro
34,597 PointsYeah, eventually I settled with Gulp as well. Here is my script, by the way, in case it helps anyone who faces the sames issues:
But before I settled with Gulp, I wasted about 3 weeks going in circles. First I used django-pipelines, which never fully worked. Then I used django-compressor. This fared much, much better, but I hit a point in development I could really use a source map to debug the scss and I couldn't make it work with django-compressor.
The only downside of Gulp is the node_modules overhead. 20Mb of codes and dependencies is madness!
Even if it's a backend web framework, it makes no sense for a framework that claims to be 'batteries-included' not to include such a vital aspect of web development. It's an ugly problem to have and to be forced to rely on Node.js, specially when you consider Rails has introduced a very elegant solution with asset pipeline 7 years ago.
Fernando Cordeiro
34,597 PointsFernando Cordeiro
34,597 PointsKyle Hartigan , that course could really use an update! Gulp 4 is very different from Gulp 3 and it fills your code with
gulp.series
andgulp-parallel
tasks!