Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial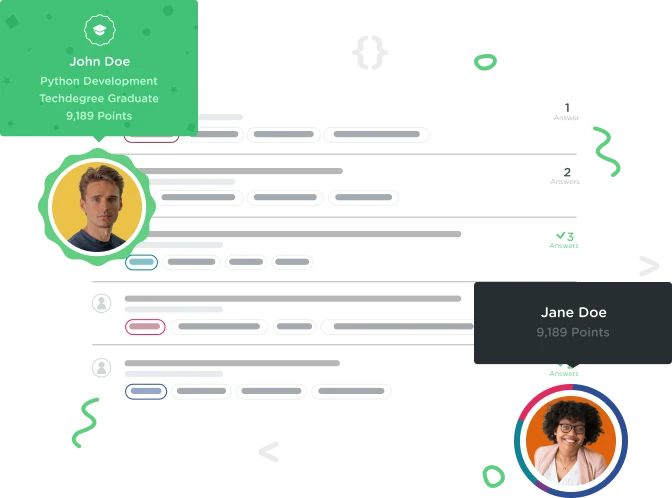

Paden Clayton
3,101 PointsUsing simple_list_item_activated_2 in Android App
First off I'm new with Java so bear with me. I am working on modifying the master detail template to list 2 lines from a dummy content file (this will later pull from shared prefs) and am not quite sure how to pull this off.
My Dummy Content class is this:
```package com.fasttracksites.skyrimjournal.data;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class JournalEntries {
/**
* An array of sample (dummy) items.
*/
public static List<JournalEntry> ITEMS = new ArrayList<JournalEntry>();
/**
* A map of sample (dummy) items, by ID.
*/
public static Map<String, JournalEntry> ITEM_MAP = new HashMap<String, JournalEntry>();
static {
// Add 3 sample items.
addItem(new JournalEntry("1", "08-30-1995", "Item 1", "Item 1 Details"));
addItem(new JournalEntry("2", "08-30-1998", "Item 2", "Item 2 Details"));
addItem(new JournalEntry("3", "08-30-2013", "Item 3", "Item 3 Details"));
}
private static void addItem(JournalEntry item) {
ITEMS.add(item);
ITEM_MAP.put(item.mId, item);
}
/**
* A dummy item representing a piece of content.
*/
public static class JournalEntry {
public String mId;
public String mDate;
public String mTitle;
public String mContent;
public JournalEntry(String id, String date, String title, String content) {
mId = id;
mDate = date;
mTitle = title;
mContent = content;
}
@Override
public String toString() {
return mContent;
}
}
}```
It is instantiated in EntryListActivity like so:
```protected static ArrayList<JournalEntry> mJournalEntries = new ArrayList<JournalEntry>(); ```
This is then being attached to the List in EntryListFragment (which is where I'm having trouble at) like so:
```String[] keys = { JournalEntries.ITEMS }
int[] ids = { android.R.id.text1, android.R.id.text2 };
SimpleAdapter adapter = new SimpleAdapter(getActivity(), EntryListActivity.mJournalEntries,
android.R.layout.simple_list_item_activated_2,
keys, ids);
setListAdapter(adapter);```
How do I properly attach the Dummy Content so that JournalEntry.mTitle is the first line and JournalEntry.mDate is the second line?
1 Answer

Jonathan Baker
2,304 PointsPaden,
You have gone the smart approach in modeling your dummy data as objects, but unfortunately SimpleAdapter is not designed to be used in this way. A SimpleAdapter takes a list of Map<String, String> objects (a mapping of string keys to string values). To achieve what your trying to do with a SimpleAdapter, the following code should work.
// Placeholder reference to an entry representation.
HashMap<String, String> entry;
// The soon to be list of entries.
List<Map<String,String>> entries = new ArrayList<Map<String, String>>();
// Configure the first entry.
entry = new HashMap<String, String>();
entry.put("title", "Item 1");
entry.put("content", "Item 1 Details");
// Add the first entry to the list.
entries.add(entry);
// Configure the second entry
entry = new HashMap<String, String>();
entry.put("title", "Item 2");
entry.put("content", "Item 2 Details");
// Add the second entry to the list.
entries.add(entry);
/*
* Configure the mapping between views and data. This first view ID will
* be used to show the value mapped to the first key in the "keys" String[].
*
* android.R.id.text1 -> "title" -> "Item 1"
* android.R.id.text2 -> "content" -> "Item 1 Details"
*/
int[] viewIDs = new int[] {
android.R.id.text1, android.R.id.text2
};
String[] keys = new String[] {
"title", "content"
};
SimpleAdapter adapter = new SimpleAdapter(getActivity(), entries, android.R.layout.simple_list_item_activated_2, keys, viewIDs);
setListAdapter(adapter);
I explain what's going on with code comments. Another approach (which I recommend) you can take in order to use your JournalEntry objects is to use an ArrayAdapter subclass instead. This allows you to simply pass in your list of JournalItems (JournalEntries.ITEMS). Then within the adapter subclass, you can define how the view is configured. Let me know if you would like to see an example of how this may look.
I hope I didn't confuse you more... ;)
-- Jonathan
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherAwesome answer. :) We cover custom ListAdapters in the upcoming Android course, and I plan on doing some sort of deep-dive as a workshop or some other non-project material in the near future.