Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial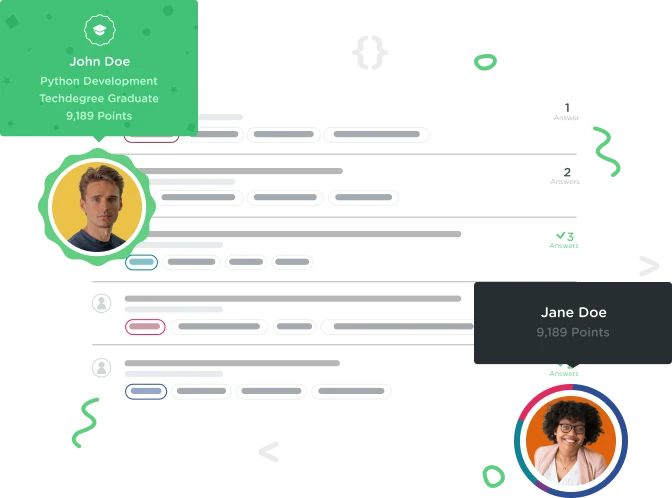
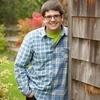
Sam Dale
6,136 PointsUsing str.format with a string that contains {}.
Hi there. I was just wondering what you need to do in order to use str.format with a string that contains {}. For example if you had the code:
print("Check {} {}".format("Here"))
Ideally I would want it to print: Check {} Here</br>
It throws an IndexError since format doesn't have enough chunks to insert into the instances of {}. But what if for some odd reason you needed to write {} in your string? Is there an escape character for { and }? I tried backslash, but that didn't work. Thanks for the help!
1 Answer
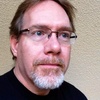
Chris Freeman
Treehouse Moderator 68,423 PointsThe .format()
method uses a special escape for braces: double-braces:
>>> "Check {{}} {}".format("Here")
'Check {} Here'
See Format String Syntax for more info
Sam Dale
6,136 PointsSam Dale
6,136 PointsAh, very interesting. Thanks!
A X
12,842 PointsA X
12,842 PointsWhew! That's a lot of braces, and so what other escape characters are there in Python besides curly braces and backslash? Could we have used backslash in this example? In other words, are there times that you want to use one escape type vs. another?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsMost of the time backslash is the escape character.
format()
is different because it may be operating on a string that has escaping for other purposes such as regex where a brace{
is the start of a range value, and\{
is a regular character, soformat
needed something different.If you had a regex that you wanted to construct using
format
the escaping can look crazy.Say I wanted a regex that matched a specific number of characters between regular braces, I could use
r'\{\w{4,}\}'
to mean four or more word characters. But if I wanted to use a variable instead of 4, I could useformat
to fill it in.First escape the existing braces
r'\{{\w{{4,}}\}}'
then insert the format field in place of the "4"
r'\{{\w{{{},}}\}}'
Then add the format method
r'\{{\w{{{},}}\}}'.format(count)
Such as