Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial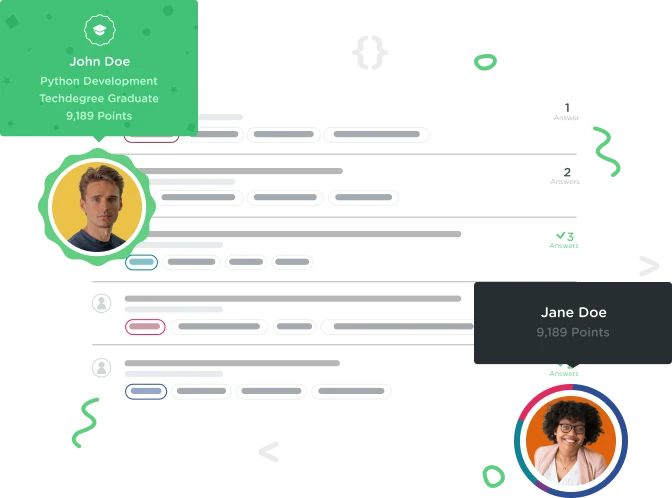

John Balladares
Courses Plus Student 9,828 PointsUsing strings as answers instead of numbers in the array quiz challenge
Hi treehouse community,
During the "Build a Quiz Challenge", I originally wrote it with both numbers and strings as acceptable answers. However, Dave only discusses how to accept numeric answers. If I wanted to accept both numbers and strings as answers would I have to use:
response = prompt(question).toString();
instead of
response = parseInt(prompt(question));
?
Thanks in advance for your help!
4 Answers
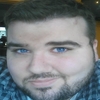
Marcus Parsons
15,719 PointsHey John,
You actually don't have to call the "toString()" method on a prompt because it returns a string, by default. All you have to do is prompt for the question. You can even go ahead and perform string based methods on a prompt i.e. here's someone yelling at you via the "toUpperCase()" method called on the prompt:
var response = prompt("What's your name?").toUpperCase();
alert(response + ", MY NAME IS INIGO MONTOYA! YOU KILLED MY FATHER! PREPARE TO DIE!");

John Balladares
Courses Plus Student 9,828 PointsMarcus Parsons thanks! That was helped make things a little bit clearer...
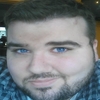
Marcus Parsons
15,719 PointsYou're very welcome, John! Good luck!
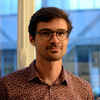
Edouard Winia
3,257 PointsHello,
I reopen this question because I am not sure of having understood. Marcus Parsons could you please clarify ?
I am not totally happy with your answer because it assumes that you know which question would expected an integer and which one would expect a string.
Would you know a way to make it more systematic ? I mean with the solution you propose you need to say to yourself "ah ok for this one I expect an integer, so let's use parseint" and "ah no for this one I expect a string, no need to use parseint.." but what if you want to be able to load a list of 100 questions which can be refresh from time to time and change, and not having to take care of the format of the expected answer ? I hope I'm clear !
Best, E.
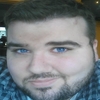
Marcus Parsons
15,719 PointsThat is a good point, Edouard. To keep it systematic, I would make every single answer a string so that you can immediately compare them, regardless of whether it is text or strictly numbers. There's no reason to use number values unless you need to do some number manipulation on that number afterwards. You wouldn't need to do that if you're doing a quiz. It's very easy to make new answers a string value regardless of where the input comes from because in almost every single input, the value you retrieve is going to be a string. Since you'll know what inputs you're going to be using, though, if one happens to return a number, you can call the "toString()" method on the numerical value and convert it to a string.
var quiz = [
["How many days are there in a standard year?", "365"],
["How many hours are there in a day?", "24"]
//etc.
];
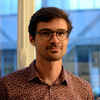
Edouard Winia
3,257 PointsHello Marcus,
Thanks, got it, indeed seems simple !
Best, E.
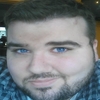
Marcus Parsons
15,719 PointsThanks for bringing that up, Edouard! Cheers!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnd to actually answer your question, what I would do is get the prompt as normal to accept strings or numbers as answers. When you need to compare the prompt received to a string, there is no problem. When you need to compare it to an integer, just run "parseInt" on the variable during the if-statement so as to preserve the content inside the variable. If you needed to compare it to a decimal, run "parseFloat" on the variable.
By keeping the variable the same, you can use the value received in some other commands like I'm doing in this very simple example below: