Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial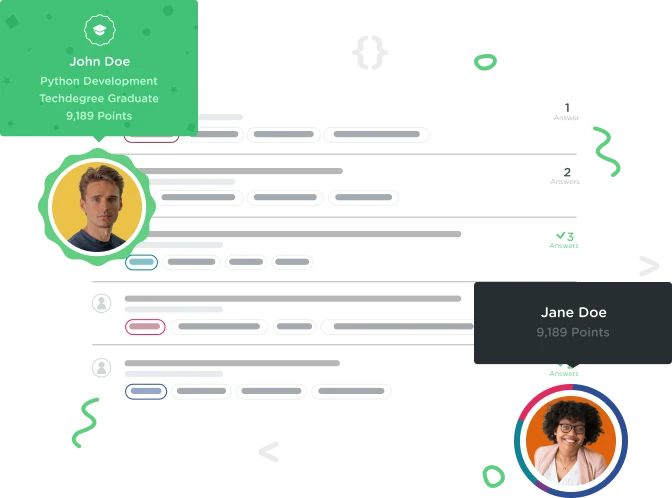
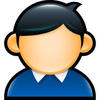
Daniel Hildreth
16,170 PointsUsing Strongly Typed Views Model Error
So in Visual Studio, my code is exactly the same as in the videos. However, my code does not produce a red squiggle under Description like it did in the video. What did I do wrong? Am I missing a particular plugin? At the bottom I have my code for all three areas where Description was changed to DescriptionHtml. Each DescriptionHtml (and the Model.Description) has an * in front it. I apologize in advance for the long list of code, and thanks for the help.
<div>@Html.Raw(Model.Description)</div>
Detail.cshtml:
@model ComicBookGallery.Models.ComicBook
@{
Layout = "~/Views/Shared/_Layout.cshtml";
ViewBag.Title = "Comic Book Detail";
}
<h1>@Model.SeriesTitle</h1>
<h2>Issue #@Model.IssueNumber</h2>
<h5>Description:</h5>
*<div>@Html.Raw(Model.Description)</div>
@if (ViewBag.Artists.Length > 0)
{
<h5>Artists:</h5>
<div>
<ul>
@foreach (var artist in ViewBag.Artists)
{
<li>@artist</li>
}
</ul>
</div>
}
ComicBookController.cs
using ComicBookGallery.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace ComicBookGallery.Controllers
{
public class ComicBooksController : Controller
{
public ActionResult Detail()
{
var comicBook = new ComicBook()
{
SeriesTitle = "The Amazing Spider-Man",
IssueNumber = 700,
*DescriptionHtml = "<p>Final issue! Witness the final hours of Doctor Octopus' life and his one, last, great act of revenge! Even if Spider-Man survives... <strong>will Peter Parker?</strong></p>",
Artists = new Artist[]
{
new Artist() { Name = "Dan Slott", Role = "Script" },
new Artist() { Name = "Humberto Ramos", Role = "Pencils" },
new Artist() { Name = "Victor Olazaba", Role = "Inks" },
new Artist() { Name = "Edgar Delgado", Role = "Colors" },
new Artist() { Name = "Chris Eliopoulos", Role = "Letters" },
}
};
return View(comicBook);
}
}
}
ComicBook.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace ComicBookGallery.Models
{
public class ComicBook
{
public int Id { get; set; }
public string SeriesTitle { get; set; }
public int IssueNumber { get; set; }
*public string DescriptionHtml { get; set; }
public Artist[] Artists { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return SeriesTitle + " #" + IssueNumber;
}
}
// series-title-issuenumber.jpg
public string CoverImageFileName
{
get
{
return SeriesTitle.Replace(" ", "-").ToLower() + IssueNumber + ".jpg";
}
}
}
}
1 Answer
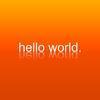
Tomasz Sporys
11,258 PointsTry rebuild the project. Sometimes it helps. The compiler needs a bit help sometimes:)
Daniel Hildreth
16,170 PointsDaniel Hildreth
16,170 PointsThat actually worked. I just copied the code from Detail.cshtml, removed previous code, and then re-pasted it. It then gave me the red squiggle and error on Description because it wasn't DescriptionHtml. Thanks.