Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial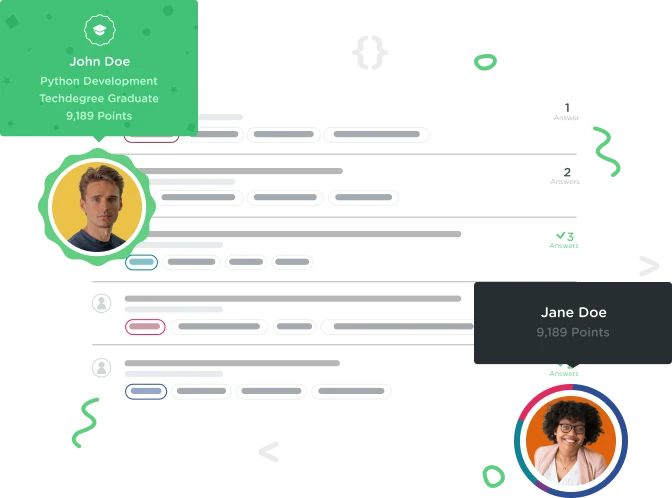
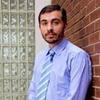
Zach Freitag
20,341 PointsUsing the filter and map methods on the todos array, create an array of unfinished task strings. - I'm lost..
I have tried:
unfinishedTask = todos
.filter( todo => `${todo.done} == false`)
.map(todo => ({todo}));
console.log(unfinishedTask);
// Returns every todo item - True & False.
I also tried:
unfinishedTask = todos
.filter( todo => `${todo.done = false}`)
.map(todo => ({todo}));
console.log(unfinishedTask);
// Returns every todo item - with their values changed to false.
I've tried a handful of other stuff with !== true and I'm getting nowhere.
Points go to best answer =)
Cheers!
const todos = [
{
todo: 'Buy apples',
done: false
},
{
todo: 'Wash car',
done: true
},
{
todo: 'Write web app',
done: false
},
{
todo: 'Read MDN page on JavaScript arrays',
done: true
},
{
todo: 'Call mom',
done: false
}
];
let unfinishedTasks;
// unfinishedTasks should be: ["Buy apples", "Write web app", "Call mom"]
// Write your code below
unfinishedTask = todos
.filter(todo => `${todo.done}` == false)
.map(todo =>`${todo.todo}`);
5 Answers
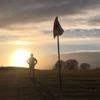
Stuart Wright
41,120 PointsThere's no need to use the template literal syntax here. Also note that you missed the s of the end of your new variable. Correct solution is:
unfinishedTasks = todos
.filter(todo => todo.done === false)
.map(todo => todo.todo);
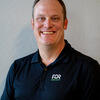
Matthew Turner
Full Stack JavaScript Techdegree Graduate 16,968 PointsI am not sure why the following isn't working? I tried in my console and it works just fine. Am I missing something?
unfinishedTasks = todos
.filter(todo => !todo.done)
.map(todo => `${todo.todo}`);

janipalomino
510 PointsBecause you don't need to use a template literal (interpolation) on your .map todo.
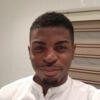
Nnanna Okoli
Front End Web Development Techdegree Graduate 19,181 PointsI was so close .. I wrote this below
unfinishedTasks = todos
.filter(todo => todo.done === false)
.map(todo => todo);
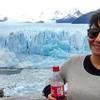
Ivonne Benites
6,044 PointsDear everybody, Good afternoon
If someone please can explain me why in the solution part where the map is placed we refer only to todo.todo ?
unfinishedTasks = todos .filter(todo => todo.done === false) .map(todo => todo.todo);
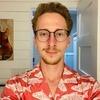
David Shulkin
Front End Web Development Techdegree Student 10,255 Pointsthe .todo
appended to todos is the objects property value which are the strings
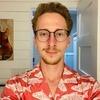
David Shulkin
Front End Web Development Techdegree Student 10,255 PointsHere I am filtering through the todos array returning 'done' tasks whose value is false. Next I map over the false todos and store them in the variable 'unfinshedTasks'
const unfinishedTasks = todos
.filter(task => task.done != true)
.map(task => task.todo)
You can also console.log() it when testing your code
.map(task => console.log(task.todo))
Zach Freitag
20,341 PointsZach Freitag
20,341 PointsThanks a ton Stuart!
I was trying to put everything together from all the videos leading up to this challenge and I thought this situation could be solved with template literals but less is more =)
Cheers!