Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial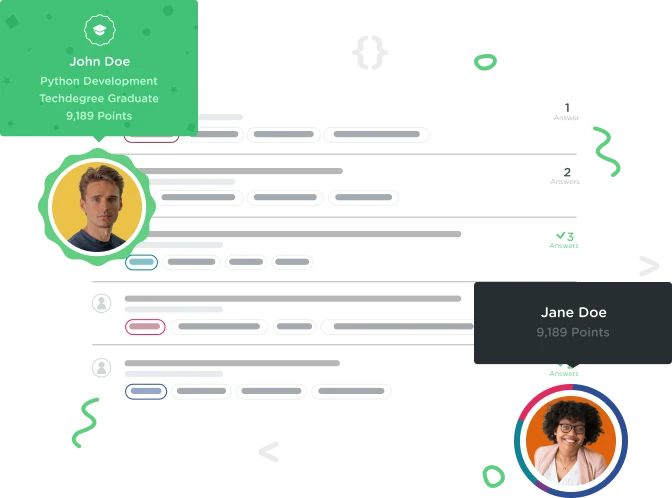
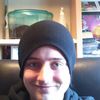
Max Reinsch
5,740 PointsUsing the internet.
Can someone please explain to me how you get from
"public boolean equals(Object anObject)
Compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.
Overrides: equals in class Object Parameters: anObject - The object to compare this String against Returns: true if the given object represents a String equivalent to this string, false otherwise See Also: compareTo(String), equalsIgnoreCase(String)"
to the following:
if (noun.equals("dork")) { console.printf("That language is not allowed. Exiting. \n\n"); System.exit(0); }
I just want to know how to interpret this kind of information; as basic as you can please. =)
Thanks in advance.
Max.
Tag: Craig Dennis
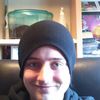
Max Reinsch
5,740 PointsThanks fbenedetti. I'm now on an online voyage of discovery. I'll let you know when I grasp what I need (wish I had more time to study).
3 Answers

Craig Dennis
Treehouse TeacherMax Reinsch , not naive at all. This is one of those things that just clicks, and then you won't even remember how you didn't get it at first. It's totally fine. It might just be a language thing.
So we can create a variable called example and store true or false in it like this:
boolean example = true;
// Or
boolean example = false;
The String.equals
method returns a true or false. So we could do this
String word = "hello";
boolean example = word.equals("hello");
The equals method should really be read as is equal to. So "hello" is equal to "hello". Then in example
would be stored the value true.
That help clear things up?
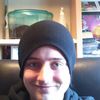
Max Reinsch
5,740 PointsYes, that's starting to make more sense now. So typically if I wanted to store a true or false value inside of a variable then "boolean example = true/false;" is how I would state that? Then, if the returned value matches that of the stored value, the code executes as planned?

Craig Dennis
Treehouse TeacherHi Max Reinsch !
First off, sorry about the confusion. Have you seen my video on Errors yet? I just re-watched this and realized that I cut a little deep when doing an edit on my script, I made a pretty big error. Whoops! I will try and fix it, thanks for pointing it out!
Can you let me know if the text below helps you please?
First, the datatype boolean
stores true or false. We use that in our conditional if
expression. The method String.equals
accepts any Object, and what I failed to mention is that String
s are an Object.
Basically I wanted to show here exploration of the documentation, you'll see that afterwards I use it to get to String.equalsIgnoreCase
through the See Also section of the documentation.
Essentially that code is this: If the word entered is equal to dork, exit the program.
Does that help?
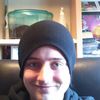
Max Reinsch
5,740 PointsI think there's a few bits of info I'm missing (either wasn't included or I havn't absorbed it all). I think the main reason I'm having difficulty with, let's say "public boolean equals(Object anObject)" is because of the order it's presented in. I'm so used to the basic "A+B=C" concept that this has thrown me off.
Forgive me for being so naive, it's all a little new to me.
I fully understand a lot of what you've said untill this tutorial.
Perhaps a basic explanation of the order of objects/syntax?
Cheers for getting back to me Craig, your tutorials are great!

Craig Dennis
Treehouse Teacherif (whateverIsInTheseParenthesisIsTrue) {
//Then this code will run
}
// This code below keeps running as normal.
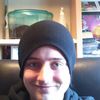
Max Reinsch
5,740 PointsStarting to make a lot more sense now. I shall put it into practice.
Cheers Craig Dennis and fbenedetti.
fbenedetti
2,010 Pointsfbenedetti
2,010 PointsGood question!
Let's look again at this line:
public boolean equals(Object anObject)
What that line is saying is that
equals
is a function that can be called anywhere (public
), and that return a true|false value (boolean
). It accepts an object as a parameter, and just one object (Object anObject
).Why this way of presenting a method, you may ask. Because a method is a function that belongs to a given type --in this case,
String
. Let's have a look at theString.java
source code for theequals
method:There you go. The source code presents the usage template right at the beginning, in the first comment. Then you get the rest of the function (which I find very readable and easy to understand). Sometimes going to the source code of the method can be enlightening.
I hope this answers your question. I am quite a beginner myself, and trying to answer your doubt made me learn a few things. :-)
PS: about the object.method notation, see The Java Dot Notation.