Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial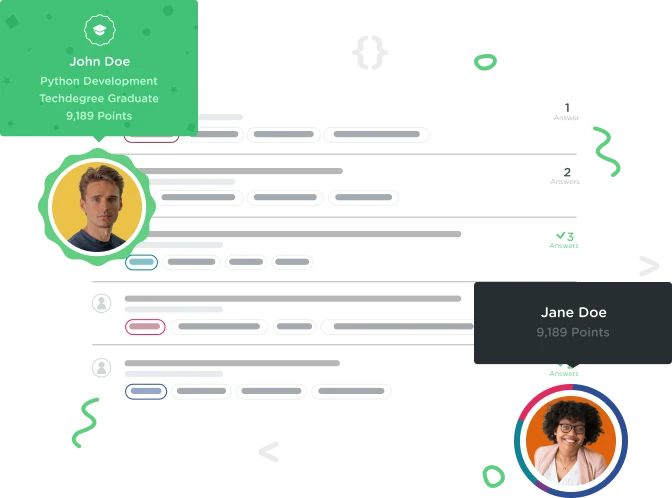

Owen Orsetti
19,102 PointsUsing the "|" operator in Django 2.0's "Path"
In this step, Kenneth has us write a URL for creating a question that includes "mc|tf" to determine the question type.
Since the urls method he uses has been depricated in Django 2.0, I have been using the path method instead, usually to similar results.
However, try as I might I cannot get the "|" part of this URL from this step to work using path.
As an alternative, you can import and use re_path instead, which allows you to use regular expressions in exactly the same way as Kenneth is using:
from django.urls import path, re_path
re_path(r'(?P<quiz_pk>\d+)/create_question/(?P<question_type>mc|tf)/$', views.create_question, name='create_question'),
So this post serves 2 purposes:
1) To anyone struggling to get path working with the "|" part of the URL, you can use r_path instead.
2) If anyone knows how to use path with "|" please let me know because I wasn't able to figure it out and I would like to know!
Cheers, Owen
1 Answer
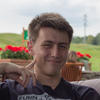
Jonatan Witoszek
15,384 PointsThere's no escaping regular expressions in this case.
However we can still use |
with path
. To do so we have to define custom path conventer like this:
class QuestionType:
regex = 'mc|tf'
def to_python(self, value):
return str(value)
def to_url(self, value):
return value
Then we have to register our conventer:
register_converter(QuestionType, 'question')
(the question
string here is what we will use in path
instead of, for example int
)
After that we can use our registered conventer in path:
path('<int:quiz_pk>/create_question/<question:question_type>',
views.create_question, name='create_question'),
Owen Orsetti
19,102 PointsOwen Orsetti
19,102 PointsNice, thanks! Seems like a bit overkill for this situation, since r_path works, but I didn't know you could create custom path converters.
Thanks! Owen