Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial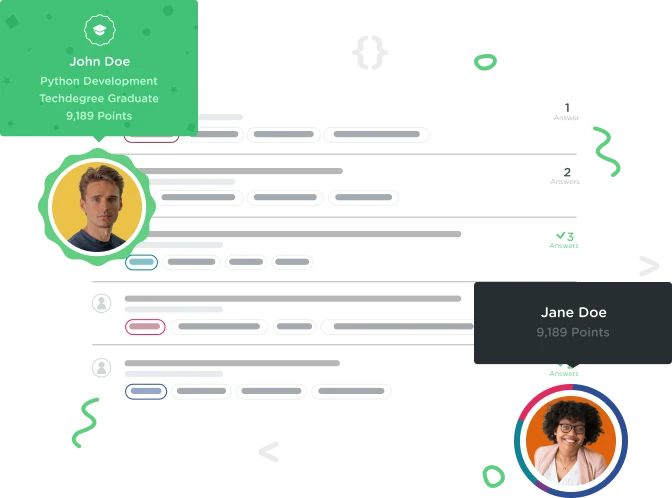

saddam lashuel
Courses Plus Student 9,178 PointsUsing the reduce method
const customers = [ { name: "Tyrone", personal: { age: 33, hobbies: ["Bicycling", "Camping"] } }, { name: "Elizabeth", personal: { age: 25, hobbies: ["Guitar", "Reading", "Gardening"] } }, { name: "Penny", personal: { age: 36, hobbies: ["Comics", "Chess", "Legos"] } } ]; let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"] // Write your code below hobbies.reduce((arr,arrf)=> [...arr, arrf.personal.[...hobbies],[]);
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies.reduce((arr,arrf)=> [...arr, arrf.personal.[...hobbies],[]);
3 Answers
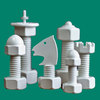
Steven Parker
231,275 PointsYou don't need bracket notation to access the "hobbies" property, that's actually the name of the property.
Also, you need to position your scatter operator at the beginning of the fully qualified name.
And you need to assign "hobbies", the "reduce" should be applied to the "customers" array.

saddam lashuel
Courses Plus Student 9,178 Points1-hobbies = customers.reduce((arr,arrf)=> [...arr, arrf.personal...hobbies,[]); 2-hobbies = customers.reduce((arr,arrf)=> [...arr, arrf.personal....hobbies,[]);

saddam lashuel
Courses Plus Student 9,178 PointsThank you so much !!!

saddam lashuel
Courses Plus Student 9,178 PointsIs this right? hobbies = customers.reduce((arr,arrf)=> [...arr, arrf.personal.hobbies...,[]);
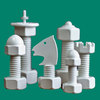
Steven Parker
231,275 PointsThe scatter operator goes in front of the term, like you have on the first one.

saddam lashuel
Courses Plus Student 9,178 Pointshobbies = customers.reduce((arr,arrf)=> [...arr, arrf.personal....hobbies,[]);
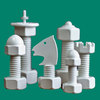
Steven Parker
231,275 PointsI mean it should go in front of the entire term (not just the property name). Plus it looks like you're missing a closing bracket ("]").

amz baz
2,053 Pointshobbies = customers.reduce( (arr,arrf) => [...arr, ...arrf.personal.hobbies], []);