Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial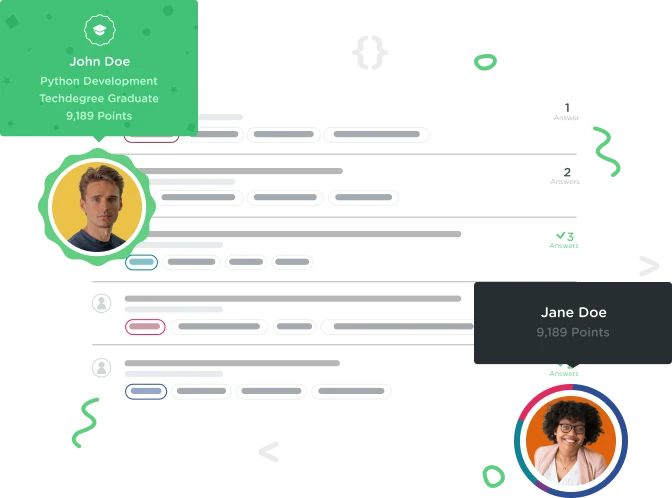
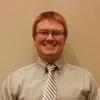
Patrick Shushereba
10,911 PointsUsing the Spaceship operator
I had a quick question regarding one of the Ruby lessons.
Here is the code from the lesson:
class Player
include Comparable
attr_accessor :name, :score
def <=>(other_player)
score <=> other_player.score
end
def initialize(name, score)
@name = name
@score = score
end
end
player1 = Player.new("Jason", 100)
player2 = Player.new("Kenneth", 80)
puts "player1 > player2: %s" % (player1 > player2)
puts "player1 < player2: %s" % (player1 < player2)
My question is when Jason goes to define the spaceship operator, he passes in other_player as an argument. How does the comparison between the two players take place when other_players isn't referenced anywhere else? How does this manage to evaluate to true?
2 Answers
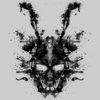
Brian O'Grady
10,094 PointsIn this section the argument is only a descriptor and not a variable. It does not need to be defined it only relates that the score of the player in question is bigger, smaller or equal to the other_player.score.
Ruby works with arguments like that.

Ulfar Ellenarson
5,277 PointsTo expand on Brian's answer the other_player as a descriptor; example would be that you could use any name you like. Try changing the parameter other_player to my_player or compare_me and it will still work.