Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial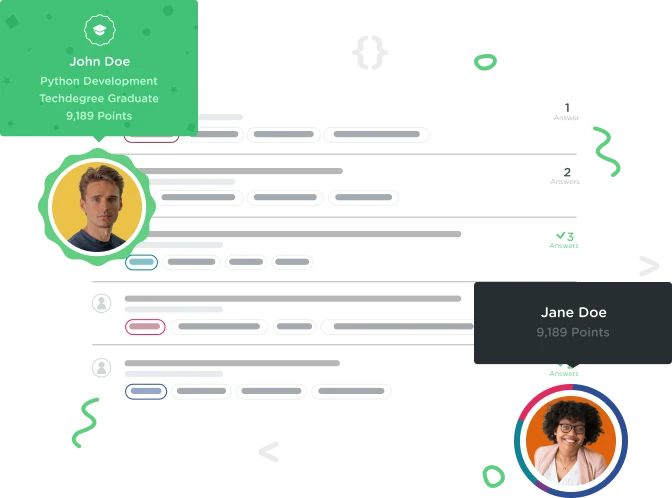

David Hilborn
15,386 PointsUsing Toasts in other classes?
After finishing the Toast notifications lesson I started playing with it to try and better get to grips with them. I wanted to try and make a Toast pop up each time the user clicked the fun facts button and say something simple such as "Try another fact!". How would you go about doing this?
I have posted the code I'm using below, I thought it would be part of the FactBook class so that each time getFact is called the Toast would be displayed as well. However I can't get a Toast to work outside of the FunFactsActivity class.
No idea if I'm just thinking about it all wrong but any help in understanding it better would be greatly appreciated!
public class FunFactsActivity extends Activity {
private FactBook mFactBook = new FactBook();
private ColorWheel mColorWheel = new ColorWheel();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign them the Views from the layout file
final TextView factLabel = (TextView)findViewById(R.id.factTextView);
final Button showFactButton = (Button)findViewById(R.id.showFactButton);
final RelativeLayout relativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
//Update the label wth the dynamic fact
factLabel.setText(fact);
int color = mColorWheel.getColor();
relativeLayout.setBackgroundColor(color);
showFactButton.setTextColor(color);
}
};
showFactButton.setOnClickListener(listener);
Toast.makeText(this, "Fun Facts has started", Toast.LENGTH_LONG).show();
}
}
import java.util.Random;
public class FactBook {
// Member variables - properties about the object
String [] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."};
// methods (Things the object can do)
public String getFact() {
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); // construct a new random number generator
int randomNumber = randomGenerator.nextInt(mFacts.length);
fact = mFacts[randomNumber];
return fact;
}
}
Thanks :)

Ben Jakuben
Treehouse TeacherGreat explanation, miguelcastro2! You should move that to an "Answer" instead of a "Comment" so it can be selected as the Best Answer. :)
1 Answer

David Hilborn
15,386 PointsAwesome thanks for the breakdown! I did wonder if it could go directly within the onClick method but wasn't sure how.
Thanks for the fast reply though, very helpful to see :)
miguelcastro2
Courses Plus Student 6,573 Pointsmiguelcastro2
Courses Plus Student 6,573 PointsThe Toast library requires a context which is essentially the current activity. Without having a reference to the current Activity the Toast command has no way of knowing where to display the message it produces. You could pass the context into your Factbook class, but it is not really necessary and it would not be good design to do so. We want to keep the Factbook focused on producing facts and our Activity can be responsible for showing the Toast messages.
To add a Toast message per each click on the fun facts button, simply add your Toast message to the onClick() method associated with your listener: