Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial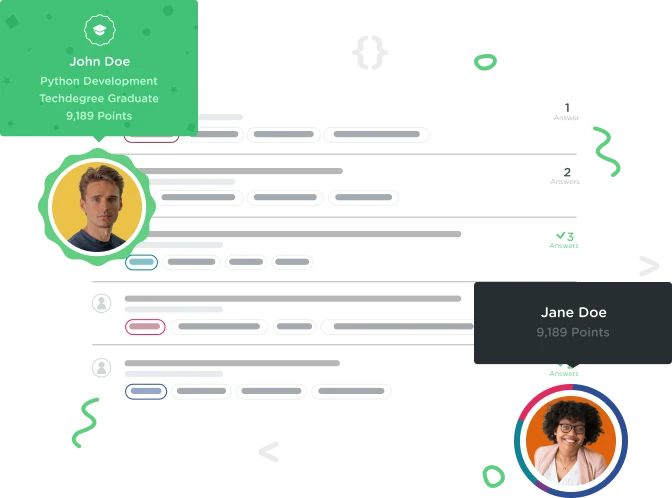

James Croxford
12,723 Points'Using try...catch and async/await in the event listener' class extension- what's the purpose of this?
So following the class, the code for establishing print outs of astronauts in space becomes:
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
// Handle all fetch requests
async function getJSON(url) {
try {
const response = await fetch(url);
return await response.json();
} catch (error) {
throw error;
}
}
async function getPeopleInSpace(url) {
const peopleJSON = await getJSON(url);
const profiles = peopleJSON.people.map( async (person) => {
const craft = person.craft;
const profileJSON = await getJSON(wikiUrl + person.name);
return {...profileJSON, craft};
});
return Promise.all(profiles);
}
// Generate the markup for each profile
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', (event) => {
event.target.textContent = "Loading...";
getPeopleInSpace(astrosUrl)
.then(generateHTML)
.catch( e => {
peopleList.innerHTML = '<h3>Something went wrong!</h3>';
console.error(e);
})
.finally( () => event.target.remove());
});
Then right at the end of the video Guil recommends having a look at the teacher's notes for implementing the following try-catch block inside of the event listener on btn:
btn.addEventListener('click', async (event) => {
event.target.textContent = 'Loading...';
try {
const astros = await getPeopleInSpace(astrosUrl);
generateHTML(astros);
} catch(e) {
astrosList.innerHTML = '<h3>Something went wrong!</h3>';
console.error(e);
} finally {
event.target.remove();
}
});
Is this here to just show us how flexible try-catch blocks are within JavaScript (i.e. they can be refactored out of a separate function into the event listener? Is there another 'lesson' to be seen here?
If it is straight up refactoring, surely it would be more sensible to leave the try-catch block within the function getJSON, to improve how modular this piece of code is, allowing developers to modify it separately to the event listener? Or put another way- what is the advantage of putting the try-catch block directly into the event listener like this?