Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial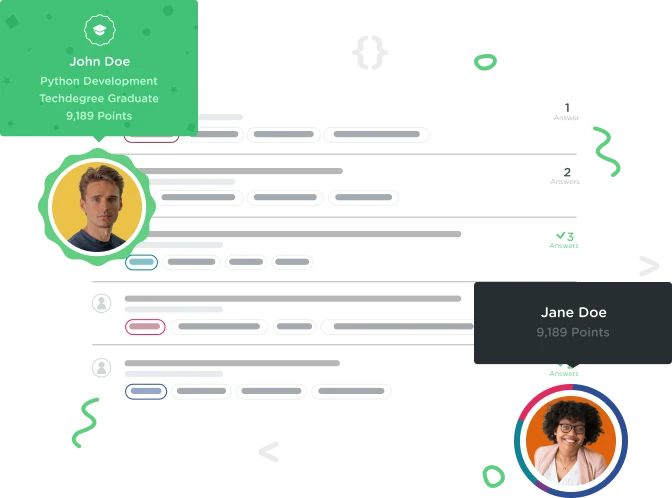

Andy McDonald
Python Development Techdegree Graduate 13,801 PointsUsing variable as attribute
I started writing the following code:
def edit():
toedit = str(input('What book do you want to edit'))
part = input('What do you want to change about it? \nType number to select follwing: \n1. Title \n2. Author\n3. Published date\n4. Price\n')
if part == '1':
change = input('Okay, what do you want to change the title to?')
bookie = models.session.query(models.Book).filter_by(title=toedit)
for bkie in bookie:
bkie.title = change
print(bkie)
And repeated this block for each attribute of the object. And then realized I could make it real dry by only writing the bottom part of the block once and throwing some variables in there like so:
elif part == '3':
part = 'pubdate'
change = input(f'Okay, who should I change the {part} to?')
bookie = models.session.query(models.Book).filter_by(part=toedit)
for bkie in bookie:
bkie.part = change
This does not work because a variable cant be passed as an attribute. Or can it? Is there any way to make this work?
1 Answer
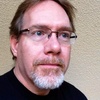
Chris Freeman
Treehouse Moderator 68,441 PointsHey Andy McDonald, excellent question!
Using star notation, a dict
can be used as keyword/pairs. If using part
as a variable, the dict can be defined then expanded as kwargs.
# define
kwdict = {part: toedit}
# use as
bookie = models.session.query(models.Book).filter_by(**kwdict)
# then
for bkie in bookie:
setattr(bkie, part, change)
Post back if you need more help. Good luck!!!
Andy McDonald
Python Development Techdegree Graduate 13,801 PointsAndy McDonald
Python Development Techdegree Graduate 13,801 PointsUnless theres something I'm missing. I dont think this is valid syntax. I need to pass this as(attribute = 'value'}. Passing a key value pair would be more like ('key' : 'pair')
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsI've created the following code. Save to a local file and run using
python <filename>
. It may help understand using the "star notation" to unpack a dictionary into attributes.outputs:
Andy McDonald
Python Development Techdegree Graduate 13,801 PointsAndy McDonald
Python Development Techdegree Graduate 13,801 PointsI just realized how to use this and this next question is totally hypothetical: Lets say there's lots of dot notation stacked onto one object. How would you use setattr to do that for the last attribute? Is it just each attribute has its own positional argument with the last argument being what it is set equal to?
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsRegarding
setattr
use, hereβs some other examples:Outputs:
Play around with this. Post back if you need more help. Good luck!!!
Andy McDonald
Python Development Techdegree Graduate 13,801 PointsAndy McDonald
Python Development Techdegree Graduate 13,801 Pointsill get back to you in 4 weeks on that one