Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial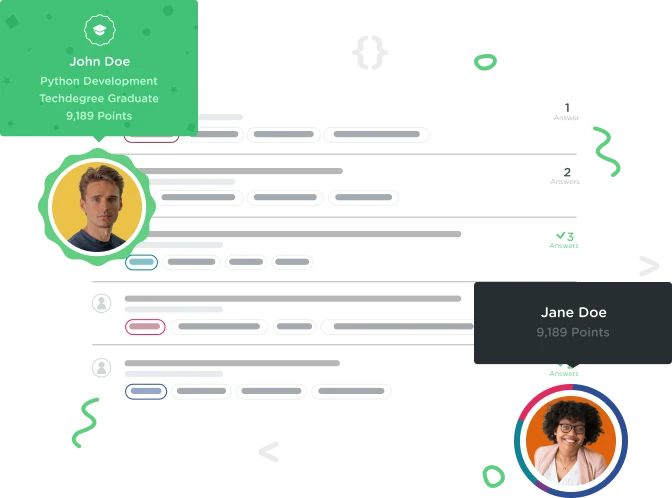

Christian Halvorsen
3,899 Pointsusing visual studio community edition, and the code is not working
Hello! I'm using Visual Studio to follow the tutorials, and it won't allow me to use double. What I mean is i've completly copy-pasted the code from the tutorial, however, if I type in 20.5 i get "That is not valid input".
Here is the code:
using System; namespace fitnessApp { class Program { static void Main() { double runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
// Prompt user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
keepGoing = false;
}
else
{
try
{
// Add minutes exercised to total
double minutes = double.Parse(entry);
if (minutes <= 0)
{
Console.WriteLine(minutes + " is not an acceptable value.");
continue;
}
else if (minutes <= 10)
{
Console.WriteLine("Better than nothing, am I right?");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go hot stuff!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja warrior in training!");
}
else
{
Console.WriteLine("Okay, now you're just showing off!");
}
runningTotal += minutes;
}
catch (FormatException)
{
Console.WriteLine("'" + entry + "' is not valid input.");
continue;
}
// Display total minutes exercised to the screen
Console.WriteLine("You've entered " + runningTotal + " minutes.");
}
// Repeat until user quits
}
Console.WriteLine("Goodbye");
Console.ReadLine();
}
}
}
Is there something going on in Visual Studio that I don't know about?
1 Answer

Edward Ries
7,388 PointsChristian, I'm not sure what could be happening but I copied and pasted your code example into Visual Studio 2015 as a new console application. Ran it and entered 20.5 and it replied with:
Way to go hot stuff! You've entered 20.5 minutes.
If I was having your problem I would debug the problem by first checking my input, for example, check the length of your input, Use a double.TryParse to test to see if it parse correctly. At this point, please try again as the code works fine without change.
Christian Halvorsen
3,899 PointsChristian Halvorsen
3,899 PointsI figured out what the issue was. My windows lang is Norwegian, so instead of using the Decimal Seperator " . " it uses " , ".
Throws an error : 20.5 Works: 20,5
I prefer working with the " . " so I found this handy snippet of code on stackoverflow:
System.Globalization.CultureInfo customCulture = (System.Globalization.CultureInfo)System.Threading.Thread.CurrentThread.CurrentCulture.Clone(); customCulture.NumberFormat.NumberDecimalSeparator = "."; System.Threading.Thread.CurrentThread.CurrentCulture = customCulture;
( Source: https://stackoverflow.com/questions/9160059/set-up-dot-instead-of-comma-in-numeric-values )