Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial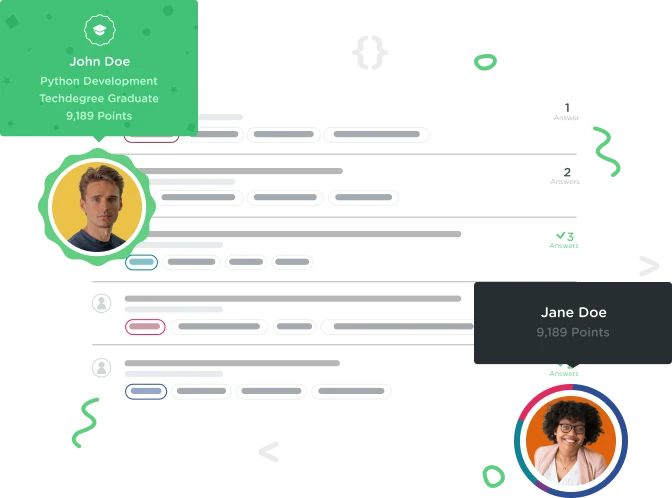

Itsa Snake
3,852 PointsUsing while correctly to continuously loop?
Hello, what would be the correct way to use 'while' in this sample code so that the user is able to continuously search?
The below works, but i highly doubt using an 'a' variable in the way i have is the 'correct' way of doing this.
docs = 'Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built-in). Tuples are also used for cases where an immutable sequence of homogeneous data is needed (such as allowing storage in a set or dict instance).'
a = 0
while(a==0):
user_word = input("Enter a random word: ")
if user_word in docs:
print(f"The word '{user_word}' is within the variable docs")
else:
print(f"the word '{user_word}' is not present")
3 Answers
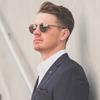
Cameron Sprague
11,272 PointsHi Itsa,
I believe I see how you are trying to use a while loop, and we call it while True. While true is tricky because it creates an infinite loop. It's ok to create an endless loop once in a while because that is how we learn. There are lots of other ways to use a while loop. I can send you a problem to solve with a while loop if you are interested in giving it a try. Just let me know. To answer your question above, please see the following code.
docs = 'Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built-in). Tuples are also used for cases where an immutable sequence of homogeneous data is needed (such as allowing storage in a set or dict instance).'
while True:
print('\n'+'Enter exit to exit the program')
user_word = input('Enter a random word: ')
if user_word == 'exit':
print('Exiting the program')
break
elif user_word in docs:
print('The word ' + user_word + ' is within the variable docs')
else:
print('the word ' + user_word + ' is not present')
It is a good idea to accompany while True with a break. Break allows a user to exit a while loop or program. You can see this in the line "if user_word == 'exit':" I am testing to see if the user has entered exit if they have then print exiting the program and break out of the loop. Please let me know if you have any questions about the code above.
As an extra point. Usually, I convert user input to uppercase or lowercase when I am checking it against values. We do not know if the user will type exit, EXIT, or eXiT. We can use either string methods of .upper or .lower. Please see the use of .lower in the code below, where I convert all of variable docs into lowercase and our user input into lowercase. Please let me know if you have any questions about the code below.
docs = 'Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built-in). Tuples are also used for cases where an immutable sequence of homogeneous data is needed (such as allowing storage in a set or dict instance).'
docs = docs.lower()
while True:
print('\n'+'Enter exit to exit the program')
user_word = (input('Enter a random word: '))
user_word = user_word.lower()
if user_word == 'exit':
print('Exiting the program')
break
elif user_word in docs:
print('The word ' + user_word + ' is within the variable docs')
else:
print('the word ' + user_word + ' is not present')

Itsa Snake
3,852 PointsThanks so much Cameron. That's helpful and brings back memories of a much earlier lesson.
As I have progressed until this stage and plan to finish all the lessons.. where you suggest I start to actually practice coding, i.e. not within workspace on treehouse! There seem to be so many different platforms like Django but I wondered where real coders usually progress to after learning the theory

Leslie Lewis
1,088 PointsI'd hardly be called a real coder (not now, anyway - that was 30 years ago!) but I find Visual Studio Code easy and helpful. It runs on my Linux and Chromebook machines, and I assume Windows too.
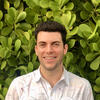
justlevy
6,326 Pointsreplit is my jam. Definitely check it out. It's a simple, web-based IDE.
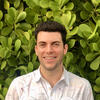
justlevy
6,326 PointsCameron Sprague - thanks for sharing that code example.
Question - why does entering 'tu' return true ('The word tu is within the variable docs'). In other words, how can we make the program return true if the entire word matches, versus a single letter?
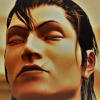
Carlton Nunes Desouza
13,447 PointsI tried splitting the docs string into a list, which then searches each individual word rather than the letters in the string:
docs = 'Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built-in). Tuples are also used for cases where an immutable sequence of homogeneous data is needed (such as allowing storage in a set or dict instance).'
docs = docs.lower()
docs_list = docs.split()
while True:
print('\n'+'Enter exit to exit the program')
user_word = input('Enter a random word: ')
user_word = user_word.lower()
if user_word == 'exit':
print('Exiting the program')
break
elif user_word in docs_list:
print(f'The word {user_word} is within the variable docs')
else:
print(f'the word {user_word} is not present')