Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial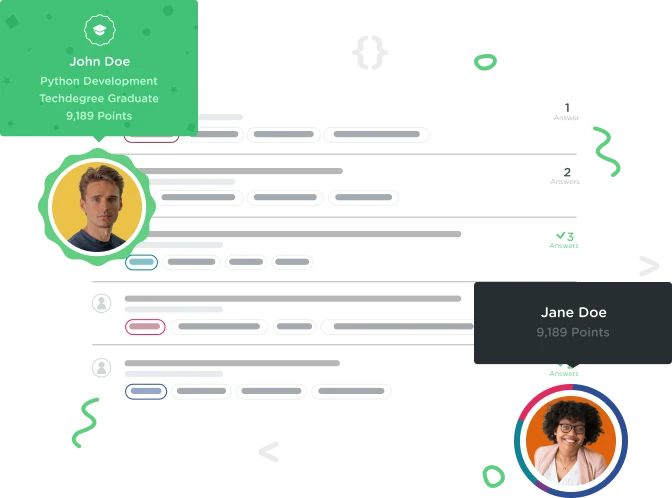
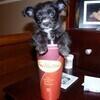
Robert Bourton
6,179 PointsUsing your own Server (Node)
The id I used for the div is ajax and btn for the button. make sure /data in app.get and in client side is the same. It can be anything and you do not need to go to this location with the address bar.
Root folder / index.html and sidebar.html should also be in the root folder /
Server Side JavaScript
server.js
const path = require('path')
const express = require('express')
const app = express()
app.use(express.static(path.join(__dirname, 'public')))
app.get('/data', (req, res, next) => {
res.sendFile(__dirname + '/sidebar.html')
})
app.get('/', (req, res, next) => {
res.sendFile(path.join(__dirname, 'index.html'))
})
app.listen(3000, ()=> {
console.log('Listening on port 3000')
})
Client Side JavaScript
/public/js/ - All javascripts files should be here. In your html file, leave off the /public/ /public/css/ - put all your css files here. /public/img/ - put all your images here. if using
script.js
const button = document.querySelector('#btn')
const div = document.querySelector('#ajax')
const xhr = new XMLHttpRequest()
xhr.onreadystatechange = () => {
if (xhr.readyState === 4 ) {
div.innerHTML = xhr.responseText
}
}
xhr.open('GET', '/data', true)
button.addEventListener('click', () => {
button.style.display = 'none'
xhr.send()
})
To make this work, you need to install Node.js and Express by NPM.