Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial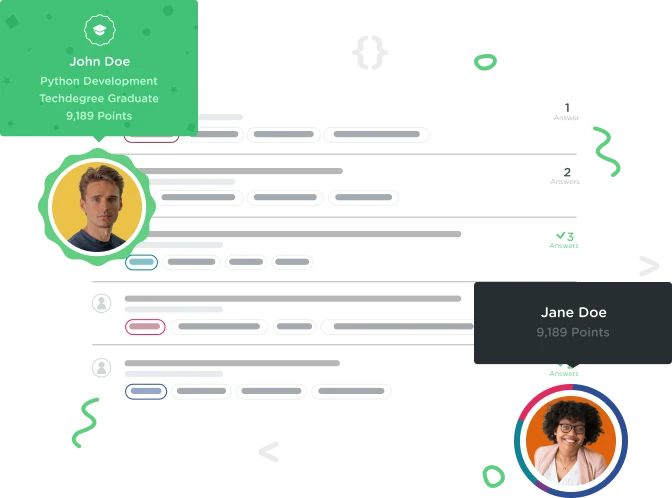

Alper Yuce
12,948 Points@Valid, doesn't work with Maven
Below, I added the model and controller java classes. Once I run, I don't receive any error. If I submit the button, it saves the empty datas. It doesn't catch the errors.
package com.test.websitesi.model;
import java.util.List;
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Lob; import javax.persistence.OneToMany; import javax.validation.constraints.NotNull;
import org.hibernate.validator.constraints.NotBlank;
@Entity public class FoodMenu {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int foodId;
@NotNull
private String name;
@NotNull
private String czDescr;
private String enDescr;
private String trDescr;
@NotNull
private int price;
private boolean isMenuOfToday;
@Lob
private byte[] bytes;
private String photoDescr;
@OneToMany
private List<ItemOrder> order;
public int getFoodId() {
return foodId;
}
public void setFoodId(int foodId) {
this.foodId = foodId;
}
public byte[] getBytes() {
return bytes;
}
public void setBytes(byte[] bytes) {
this.bytes = bytes;
}
public String getPhotoDescr() {
return photoDescr;
}
public void setPhotoDescr(String photoDescr) {
this.photoDescr = photoDescr;
}
public int getId() {
return foodId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCzDescr() {
return czDescr;
}
public void setCzDescr(String czDescr) {
this.czDescr = czDescr;
}
public String getEnDescr() {
return enDescr;
}
public void setEnDescr(String enDescr) {
this.enDescr = enDescr;
}
public String getTrDescr() {
return trDescr;
}
public void setTrDescr(String trDescr) {
this.trDescr = trDescr;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public boolean isMenuOfToday() {
return isMenuOfToday;
}
public void setMenuOfToday(boolean isMenuOfToday) {
this.isMenuOfToday = isMenuOfToday;
}
}
package com.anatoliakebap.websitesi.controller;
import java.util.List;
import javax.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.validation.BindingResult; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod;
import com.test.websitesi.model.FoodMenu; import com.test.websitesi.service.FoodMenuService;
@Controller public class FoodMenuController {
@Autowired
private FoodMenuService foodMenuService;
// Form for adding a new menu
@RequestMapping("/addmenuform")
public String formNewMenu (Model model){
model.addAttribute("foodMenu", new FoodMenu());
return "/addmenuform";
}
//save menu and retunr listmenu
@RequestMapping(value = "/addmenuform", method=RequestMethod.POST)
public String formNewMenu (@Valid FoodMenu foodMenu, BindingResult result){
if(result.hasErrors()){
return "redirect:/addmenuform";
}
foodMenuService.save(foodMenu);
return "redirect:/listmenu";
}
@SuppressWarnings("unchecked")
@RequestMapping("/listmenu")
public String listAllMenu (Model model){
List<FoodMenu> foodMenues = foodMenuService.findAll() ;
model.addAttribute("foodMenues", foodMenues);
return "/listmenu";
}
}
Here are the pom dependencies
<dependencies> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-thymeleaf --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> <version>1.5.0.RELEASE</version> </dependency> <!-- https://mvnrepository.com/artifact/org.hashids/hashids --> <dependency> <groupId>org.hashids</groupId> <artifactId>hashids</artifactId> <version>1.0.1</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework/spring-orm --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>4.3.6.RELEASE</version> </dependency> <!-- https://mvnrepository.com/artifact/org.hibernate/hibernate-core --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>5.2.6.Final</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.tomcat/tomcat-dbcp --> <dependency> <groupId>org.apache.tomcat</groupId> <artifactId>tomcat-dbcp</artifactId> <version>8.5.8</version> </dependency> <!-- https://mvnrepository.com/artifact/com.h2database/h2 --> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <version>1.4.193</version> </dependency> <!-- https://mvnrepository.com/artifact/org.hibernate/hibernate-validator --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> <version>5.4.0.Final</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies>
2 Answers
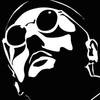
Jan Bičan
19,777 PointsI had the same problem.
After I added this dependency, it worked:
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
</dependency>
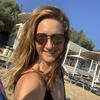
Maira Kotsovoulou
2,146 PointsAnd with Gradle I had to add: compile group: 'org.hibernate', name: 'hibernate-validator', version: '6.1.2.Final' in my dependencies for the @Valid to work