Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial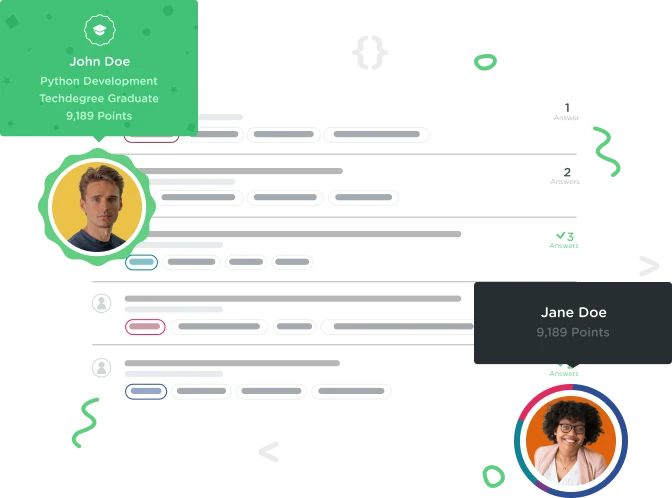
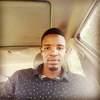
Savious Munyaradzi Ngundu
16,920 Pointsvalidate 2 fields
im stuck
from rest_framework import serializers
from . import models
class GameSerializer(serializers.ModelSerializer):
class Meta:
fields = ('id', 'name')
model = models.Game
class PlayerSerializer(serializers.ModelSerializer):
class Meta:
fields = ('id', 'name', 'age', 'parent_approval')
model = models.Player
def validate_age(self, value):
if value >= 13:
return value
raise serializers.ValidationError(
'Player age must be over 13')
class ScoreSerializer(serializers.ModelSerializer):
game = serializers.HyperlinkedRelatedField(
read_only=True, view_name='apiv2:game-detail')
player = serializers.HyperlinkedRelatedField(
read_only=True, view_name='apiv2:player-detail')
class Meta:
fields = ('id', 'game', 'player', 'score')
model = models.Score
1 Answer

diogorferreira
19,363 PointsHey Savious, I'd recommend reading the docs and going to the section labeled Object Level Validation
, it allows you to validate multiple fields, which is needed for this challenge.
- First, you'd create a validate method, that takes
self
anddata
(data being all the values passed in) - Then check whether the player is less than 13 and has
parent_approval
- If they don't then raise a
serializers.ValidationError()
with "Children under 13 require parental approval" - Otherwise, just return the data passed into the validation method
Hopefully you can solve it with that, if you need any more help then the code below is an example of what it could look like.
class PlayerSerializer(serializers.ModelSerializer):
class Meta:
fields = ('id', 'name', 'age', 'parent_approval')
model = models.Player
def validate(self, data):
if data['age'] < 13 and not data['parent_approval']:
raise serializers.ValidationError('Children under 13 require parental approval')
return data
Hope it helps!