Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial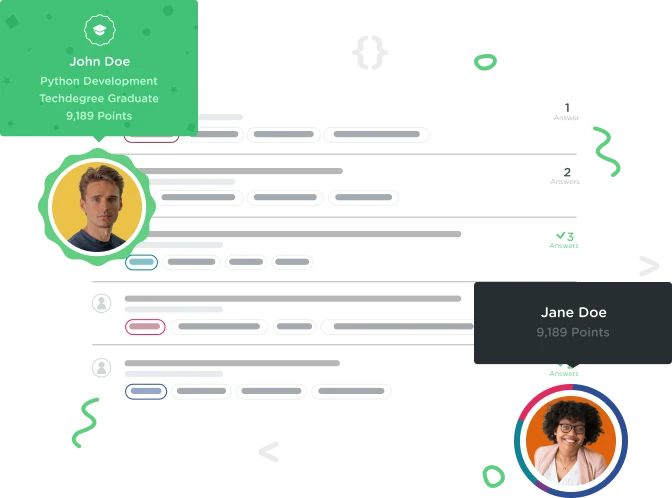
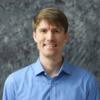
Nathan Magyar
11,332 PointsValidate Age Field in Serializer
In this challenge I'm supposed to "add a method to the Player serializer to validate the age field. If the player is younger than 13, throw a ValidationError with a message of 'Player age must be 13 or over' ".
When I run the attached code I get an error saying that a young player was accepted. Where is my mistake? Thanks for the help.
from rest_framework import serializers
from . import models
class GameSerializer(serializers.ModelSerializer):
class Meta:
fields = ('id', 'name')
model = models.Game
class PlayerSerializer(serializers.ModelSerializer):
class Meta:
fields = ('id', 'name', 'age')
model = models.Player
def validate_age(self, value):
if value >= 13:
return value
raise serializers.ValidationError(
'Player must be 13 or over')
class ScoreSerializer(serializers.ModelSerializer):
game = serializers.HyperlinkedRelatedField(
read_only=True, view_name='apiv2:game-detail')
player = serializers.HyperlinkedRelatedField(
read_only=True, view_name='apiv2:player-detail')
class Meta:
fields = ('id', 'game', 'player', 'score')
model = models.Score
1 Answer
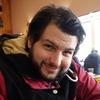
Eric M
11,546 PointsHi Nathan,
Your program logic is correct, the error message you are getting is misleading. The error is shown because the exact conditions it expects when an age of under 13 is passed are not being met.
While you are correctly raising an exception in this circumstance, you are not giving the instructed error message. This means when a <13 age is passed, the challenge checks to see if the expected error message is raised, sees a different error message and lets you know something is wrong when a <13 age is passed. It is unfortunate that it is worded as "a young player was accepted" because this is not quite what has gone wrong.
You have written 'Player must be 13 or over', the challenge is looking for 'Player age must be 13 or over'.
This is the only change you need to make.
Cheers,
Eric
Nathan Magyar
11,332 PointsNathan Magyar
11,332 PointsThank you, Eric McKibbin! 'Crazy how we can read instructions and our own code over and over again and not see silly mistakes like that. 'Much appreciated.