Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial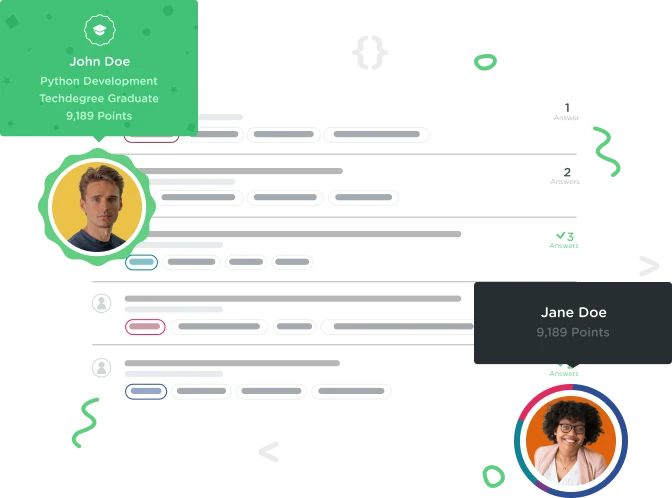
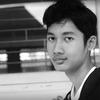
Fadli Hidayatullah
2,691 PointsValidate Email Address?
Could you give me simple example how to validate email address inside a string and validate it using regular expressions?
2 Answers
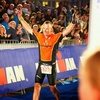
Steve Hunter
57,712 PointsHi there,
Apologies; I had assumed you were using Ruby on Rails and were trying to validate an email address inside a User model. That's not what you're doing so the validates
method is undefined. Sorry for the confusion!
Here's some working Ruby that uses the regex to match an email address. This works similarly to the above code. It defines a constant that contains the regex. I then used a string to hold an email address-type format - you wouldn probably want to verify a form-field or other user-input rather than coding the email address! Next, the =~
mthod of the Regexp
class is used to compare a string and a regular expression.
VALID_EMAIL_REGEX = /\A[\w+\-.]+@[a-z\d\-]+(\.[a-z\d\-]+)*\.[a-z]+\z/i
my_email = "my_email@gmail.com"
if( my_email =~ VALID_EMAIL_REGEX)
puts 'Matches!'
else
puts 'No match!'
end
I hope that helps you ... if not, let me know what you're trying to do and I'll see if I can help.
Steve.
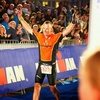
Steve Hunter
57,712 PointsHi there,
I do hope this helps.
Here, I've copied some code from the Michael Hartl tutorial (Google him!) as this code does what you're asking about.
First, it sets a constant that contains the regular expression to validate an email. Then, that constant is included within the validation of a user email within the model. It also confirms that there is an email address, that it is not more than 75 characters long and is unique across all users, but you can omit those bits.
I hope that makes sense.
Steve
VALID_EMAIL_REGEX = /\A[\w+\-.]+@[a-z\d\-]+(\.[a-z\d\-]+)*\.[a-z]+\z/i
validates :email, presence: true,
length: { maximum: 75 },
format: { with: VALID_EMAIL_REGEX },
uniqueness: { case_sensitive: false }
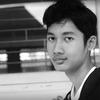
Fadli Hidayatullah
2,691 Pointssorry, but i still confusing about what should i do with the codes above. Thanks before hand that you've answered this, but in example i have the code :
email = "fadlihidayatullah.teamtreehouse@gmail.com"
VALID_EMAIL_REGEX = /\A[\w+\-.]+@[a-z\d\-]+(\.[a-z\d\-]+)*\.[a-z]+\z/i
validates :email, presence: true,
length: { maximum: 75 },
format: { with: VALID_EMAIL_REGEX },
uniqueness: { case_sensitive: false }
So, what should i do next? when i run the codes, it returns an error, what is called
undefined method `validates' for #<Context:0x00000001afd790>
(repl):3:in `initialize'