Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial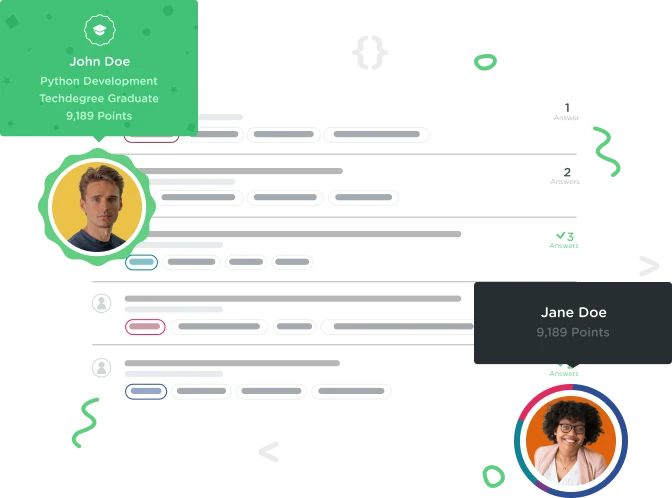
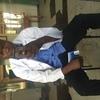
Taurai Valentine Maputsa
16,213 Pointsvalidate message for 0 tacos
Fill out the files with your finished code to pass the code challenge. Be sure to remove any app.run() calls.
from flask import Flask, g, render_template, flash, redirect, url_for, request
from flask_login import LoginManager, login_user, logout_user, login_required, current_user
from flask_bcrypt import check_password_hash
import forms
import models
app = Flask(__name__)
app.secret_key = '234LWKJEr209U)(@r029fjpJ@)euf2fp2ej'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def user_loader(userid):
try:
return models.User.get(models.User.id == userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
# connect to the database
g.db = models.database
g.db.connect()
@app.after_request
def after_request(response):
# close the db connection
g.db.close()
return response
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.select().where(
models.User.email**form.email.data
).get()
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You're now logged in!")
return redirect(url_for('index'))
else:
flash("Email or password is invalid")
except models.DoesNotExist:
flash("Email or password is invalid")
return render_template('login.html', form=form)
@app.route('/logout')
@login_required
def logout():
logout_user()
flash('You have been logged out','success')
return redirect(url_for('index'))
@app.route('/register',methods=['GET','POST'])
def register():
form = forms.RegisterForm()
if form.validate_on_submit():
flash('You are registered!','success')
models.User.create_user(
email=form.email.data,
password=form.password.data
)
return redirect(url_for('index'))
return render_template('register.html', form=form)
@app.route('/taco',methods=['GET','POST'])
@login_required
def taco():
form = forms.TacoForm()
if request.method == 'POST':
models.Taco.create(
user=current_user._get_current_object(),
protein=form.protein.data,
shell=form.shell.data,
cheese=form.cheese.data,
extras=form.extras.data
)
flash('Taco created!','success')
return redirect(url_for('index'))
return render_template('taco.html',form=form)
@app.route('/')
def index():
tacos = models.Taco.select().limit(15)
return render_template('index.html', tacos=tacos)
if __name__ == "__main__":
models.initialize()
from peewee import *
from flask_login import UserMixin
from flask_bcrypt import generate_password_hash
database = SqliteDatabase('taco.db')
class BaseModel(Model):
class Meta:
database = database
class User(UserMixin, BaseModel):
email = CharField(unique=True)
password = CharField(max_length=20)
@classmethod
def create_user(cls, email, password):
try:
cls.create(
email=email,
password=generate_password_hash(password)
)
except IntegrityError:
raise ValueError("User already exists")
class Taco(BaseModel):
user = ForeignKeyField(User)
protein = CharField()
shell = CharField()
cheese = BooleanField()
extras = CharField()
@classmethod
def create_taco(cls, user, protein, shell, cheese, extras):
cls.create(
user=user,
protein=protein,
shell=shell,
cheese=cheese,
extras=extras
)
def initialize():
database.connect()
database.create_tables([User,Taco],safe=True)
database.close()
from flask_wtf import Form
from wtforms import StringField, PasswordField, BooleanField, TextField
from wtforms.validators import DataRequired, Regexp, ValidationError, Length, EqualTo, Email
from models import User
def name_exists(form, field):
if User.select().where(User.username == field.data).exists():
raise ValidationError('User with that name already exists')
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists')
class RegisterForm(Form):
email = StringField(
'email',
validators=[
DataRequired(),
Email(),
email_exists
]
)
password = PasswordField(
'password',
validators=[
DataRequired(),
Length(min=2),
EqualTo('password2', message='Passwords must match')
]
)
password2 = PasswordField(
'Confirm password',
validators=[DataRequired()]
)
class LoginForm(Form):
email = StringField(
'email',
validators=[
DataRequired(),
Email()
]
)
password = PasswordField(
'password',
validators=[
DataRequired()
]
)
class TacoForm(Form):
protein = StringField(
'protein',
validators=[DataRequired()]
)
shell = StringField(
'shell',
validators=[DataRequired()]
)
cheese = BooleanField(
'cheese',
validators=[DataRequired()]
)
extras = StringField(
'extras',
validators=[DataRequired(),Length(100)]
)
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="message">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
{% if current_user.is_authenticated() %}
<a href="{{ url_for('logout') }}">log out</a>
<a href="{{ url_for('taco') }}">add a new taco</a>
{% else %}
<a href="{{ url_for('register') }}">sign up</a>
<a href="{{ url_for('login') }}">log in</a>
{% endif %}
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<!-- taco attributes here -->
{% endfor %}
</tbody>
</table>
{% else %}
<!-- message for missing tacos -->
{% endif %}
{% endblock %}
1 Answer
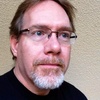
Chris Freeman
Treehouse Moderator 68,441 PointsYou are very close. In the file templates/index.html
There place for a message for missing tacos:
<!-- message for missing tacos -->
Replace this with the appropriate message and you'll be back on track.
Post back if you need more help. Good luck!!
Taurai Valentine Maputsa
16,213 PointsTaurai Valentine Maputsa
16,213 Pointshelp i keep getting this "validate message for 0 tacos"